python如何读取fasta序列文件,然后计算密码子个数
时间: 2023-07-18 13:11:03 浏览: 144
你可以使用Biopython库来读取fasta格式的序列文件,然后使用正则表达式来计算密码子个数。
以下是一个示例代码,假设你的fasta文件名为"sequence.fasta":
```python
from Bio import SeqIO
import re
# 读取fasta文件
for seq_record in SeqIO.parse("sequence.fasta", "fasta"):
sequence = str(seq_record.seq)
# 计算密码子个数
codon_count = {}
for i in range(0, len(sequence), 3):
codon = sequence[i:i+3]
if re.match("^[ATCG]+$", codon): # 确保是合法的碱基序列
codon_count[codon] = codon_count.get(codon, 0) + 1
print(codon_count)
```
这个代码会输出一个字典,其中键是密码子,值是密码子的个数。
相关问题
安装Biopython模块,并利用该模块将 NC_045512_N.fasta 文件中的序列翻译成氨基酸序列,并输出为fasta格式文件。
安装 Biopython 模块通常需要通过 Python 的包管理器 pip 进行。首先,确保已经安装了 pip,然后可以运行以下命令来安装:
```bash
pip install biopython
```
如果你的系统中还没有 pip,可以先安装它,例如在 Ubuntu 或 Debian 中:
```bash
sudo apt-get update
sudo apt-get install python3-pip
```
接下来,我们可以使用 Biopython 的 SeqIO 和.translate 函数来读取并翻译 FASTA 文件中的 DNA 序列。这里是一个简单的例子:
```python
from Bio import SeqIO
# 读取 fasta 文件
with open("NC_045512_N.fasta", "r") as handle:
record = SeqIO.read(handle, "fasta")
# 获取序列并翻译成蛋白质
dna_seq = str(record.seq)
protein_seq = record.seq.translate()
# 将翻译后的序列保存到新的 FASTA 文件
output_handle = open("translated_protein.fasta", "w")
output_handle.write(">Translated protein sequence\n")
output_handle.write(protein_seq.translate(to_stop=True).seq + "\n") # 使用 to_stop=True 结束在第一个终止密码子处
output_handle.close()
```
这将会创建一个新的名为 "translated_protein.fasta" 的文件,其中包含转录的氨基酸序列。
f=open('D:/QLNU/writing/homework/BI/2022-2023第二学期作业/20230607/hORFeome_V8.1.fasta','r+') f_out=open('D:/QLNU/writing/homework/BI/2022-2023第二学期作业/20230607/out.CSV','w+') global codon_counts codon_counts = {} # DNA序列 lines=f.readlines() if lines[0]=='>': pass else: dna_sequence = lines # 将序列分割成三个一组的密码子 codons = [dna_sequence[i:i+3] for i in range(0, len(dna_sequence), 3)] # 统计每个密码子出现的次数 for codon in codons: if codon in codon_counts: codon_counts[codon] += 1 else: codon_counts[codon] = 1 # 计算每个密码子的使用频率 total_codons = len(codons) codon_frequencies = {} for codon in codon_counts: codon_frequencies[codon] = codon_counts[codon] / total_codons # 输出结果 f.write(codon_frequencies)优化这段代码
Sure, I can help you optimize the code. Here are some suggestions:
1. Use "with open" to handle file I/O instead of "open" and "close". This ensures that the file is properly closed even if an exception is raised.
2. Use a dictionary comprehension to count the occurrences of each codon.
3. Use a generator expression to calculate the total number of codons.
4. Use the csv module to write the results to the output file.
Here's the optimized code:
```python
import csv
codon_counts = {}
with open('D:/QLNU/writing/homework/BI/2022-2023第二学期作业/20230607/hORFeome_V8.1.fasta', 'r') as f:
dna_sequence = f.read().replace('\n', '')
# count the occurrences of each codon
codon_counts = {dna_sequence[i:i+3]: codon_counts.get(dna_sequence[i:i+3], 0) + 1 for i in range(0, len(dna_sequence), 3)}
# calculate the total number of codons
total_codons = sum(codon_counts.values())
codon_frequencies = {}
for codon in codon_counts:
codon_frequencies[codon] = codon_counts[codon] / total_codons
# write the results to the output file
with open('D:/QLNU/writing/homework/BI/2022-2023第二学期作业/20230607/out.CSV', 'w', newline='') as f_out:
writer = csv.writer(f_out)
writer.writerow(['Codon', 'Frequency'])
for codon in codon_frequencies:
writer.writerow([codon, codon_frequencies[codon]])
```
This code reads in the DNA sequence from the input file, counts the occurrences of each codon using a dictionary comprehension, calculates the total number of codons using a generator expression, calculates the frequency of each codon, and writes the results to the output file using the csv module.
阅读全文
相关推荐
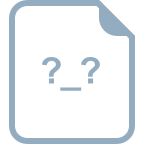
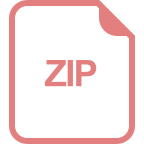
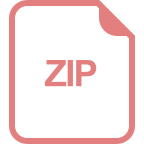
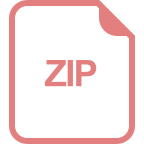
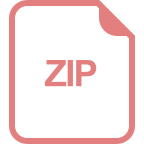
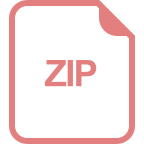
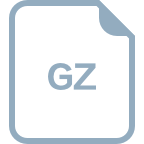
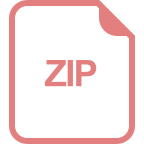
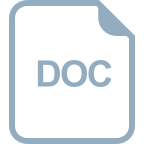
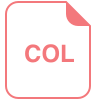
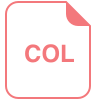
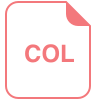

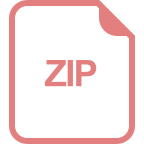
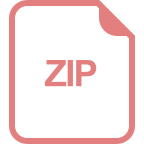