tensor.view(batch_size, -1)是什么意思
时间: 2024-05-16 20:13:53 浏览: 14
在PyTorch中,`tensor.view(batch_size, -1)`的作用是将一个tensor的形状改变为指定的形状`(batch_size, -1)`。
其中`batch_size`是指定的batch大小,而`-1`表示该维度的大小应该被自动计算,以保持原有tensor中元素数量不变。这样做的好处是,我们可以根据batch大小动态地调整tensor的shape,而不用手动计算每个维度的大小。
例如,假设我们有一个形状为`(4, 5, 6)`的tensor,我们想将它变成一个形状为`(2, -1)`的tensor,这样就能将前两维压缩成一个维度,并且自动计算出新的tensor中第二个维度的大小。具体操作如下:
``` python
import torch
# 创建一个形状为(4, 5, 6)的tensor
x = torch.randn(4, 5, 6)
# 将前两维压缩成一个维度,第二个维度的大小会自动计算
x = x.view(2, -1)
print(x.shape) # 输出: torch.Size([2, 60])
```
这里的`-1`会被自动计算为`60`,即原有tensor中剩余维度的元素数量。
相关问题
import torchimport torch.nn as nnclass MultiHeadAttention(nn.Module): def __init__(self, d_model, num_heads): super(MultiHeadAttention, self).__init__() self.num_heads = num_heads self.d_model = d_model assert d_model % self.num_heads == 0 self.depth = d_model // self.num_heads self.Wq = nn.Linear(d_model, d_model) self.Wk = nn.Linear(d_model, d_model) self.Wv = nn.Linear(d_model, d_model) self.fc = nn.Linear(d_model, d_model) def scaled_dot_product_attention(self, Q, K, V, mask=None): d_k = Q.size(-1) scores = torch.matmul(Q, K.transpose(-1, -2)) / torch.sqrt(torch.tensor(d_k, dtype=torch.float32)) if mask is not None: scores = scores.masked_fill(mask == 0, -1e9) attention = torch.softmax(scores, dim=-1) output = torch.matmul(attention, V) return output, attention def split_heads(self, x, batch_size): x = x.view(batch_size, -1, self.num_heads, self.depth) return x.permute(0, 2, 1, 3) def forward(self, Q, K, V, mask=None): batch_size = Q.size(0) Q = self.Wq(Q) K = self.Wk(K) V = self.Wv(V) Q = self.split_heads(Q, batch_size) K = self.split_heads(K, batch_size) V = self.split_heads(V, batch_size) scaled_attention, attention = self.scaled_dot_product_attention(Q, K, V, mask) scaled_attention = scaled_attention.permute(0, 2, 1, 3).contiguous() scaled_attention = scaled_attention.view(batch_size, -1, self.d_model) output = self.fc(scaled_attention) return output, attention
上述代码是一个用PyTorch实现的多头注意力机制(Multi-Head Attention)的模块,该模块可以被用来构建神经网络模型。它的参数有:
- d_model:表示输入向量的维度,也就是embedding的维度。
- num_heads:表示attention头的数量。
输入的维度是:
- Q, K, V:三个输入张量的维度都为 [batch_size, seq_length, d_model],其中batch_size代表batch的大小,seq_length代表输入序列的长度,d_model代表输入向量的维度。
- mask:一个shape为[batch_size, 1, seq_length, seq_length]的张量,用于掩盖无效的位置,使得在计算注意力矩阵时,注意力矩阵中无效位置对应的权重为0。如果没有无效位置,则可以设置为None。
import torch import torch.nn as nn import numpy as np from torch.utils.data import DataLoader, TensorDataset import pandas as pd class RNN(nn.Module): def init(self, input_size, hidden_size, output_size): super(RNN, self).init() self.hidden_size = hidden_size self.rnn = nn.RNN(input_size, hidden_size, num_layers=1, batch_first=True) self.fc = nn.Linear(hidden_size, output_size) def forward(self, x): h0 = torch.zeros(1, x.size(0), self.hidden_size) out, _ = self.rnn(x, h0) out = self.fc(out[:, -1, :]) return out input_size = 1 hidden_size = 32 output_size = 1 model = RNN(input_size, hidden_size, output_size) criterion = nn.MSELoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.001) df = pd.read_csv('beijing_wangjing_125_new.csv') congestion_index = df['idx'].values congestion_index_tensor= torch.tensor(congestion_index, dtype=torch.float32).view(-1,1,1) print(congestion_index_tensor) for epoch in range(100): outputs = model(congestion_index_tensor) loss = criterion(outputs, congestion_index_tensor) optimizer.zero_grad() loss.backward() optimizer.step()代码如何修改初始隐藏层状态为3
要将初始隐藏层状态设置为3,需要在RNN类的__init__函数中增加一个参数,并在forward函数中使用该参数来初始化隐藏层状态。具体修改如下:
```
class RNN(nn.Module):
def __init__(self, input_size, hidden_size, output_size, num_layers):
super(RNN, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.rnn = nn.RNN(input_size, hidden_size, num_layers=num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x, h=None):
if h is None:
h = torch.zeros(self.num_layers, x.size(0), self.hidden_size)
out, h = self.rnn(x, h)
out = self.fc(out[:, -1, :])
return out, h
input_size = 1
hidden_size = 32
output_size = 1
num_layers = 1
model = RNN(input_size, hidden_size, output_size, num_layers)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
df = pd.read_csv('beijing_wangjing_125_new.csv')
congestion_index = df['idx'].values
congestion_index_tensor = torch.tensor(congestion_index, dtype=torch.float32).view(-1, 1, 1)
h = torch.zeros(num_layers, 1, hidden_size) + 3 # 初始隐藏层状态设置为3
for epoch in range(100):
outputs, h = model(congestion_index_tensor, h)
loss = criterion(outputs, congestion_index_tensor)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
在该代码中,我们增加了一个num_layers参数,用于指定RNN层的层数。在forward函数中,我们增加了一个h参数,用于初始化隐藏层状态,同时在第一次调用forward函数时,如果h参数为None,则将其初始化为全零张量。在训练过程中,我们通过将h参数设置为一个值为3的全零张量,来将初始隐藏层状态设置为3。
相关推荐
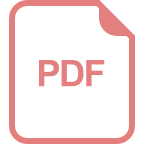












