用python# 3.以点(Point)类为基类,重新定义矩形类和圆类。点为直角坐标点,矩形水平放置,由左下方的顶点和长宽定义。 # 圆由圆心和半径定义。派生类操作判断任一坐标点是在图形内,还是在图形的边缘上,还是在图形外。编程测试类设计是否正确。
时间: 2024-02-25 20:56:15 浏览: 80
好的,我会帮你解答这个问题。以下是代码实现,你可以参考一下:
```python
import math
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
class Rectangle(Point):
def __init__(self, x=0, y=0, width=0, height=0):
super().__init__(x, y)
self.width = width
self.height = height
def is_inside(self, point):
if (self.x <= point.x <= self.x + self.width) and (self.y <= point.y <= self.y + self.height):
return True
elif (self.x == point.x and self.y <= point.y <= self.y + self.height) or \
(self.x + self.width == point.x and self.y <= point.y <= self.y + self.height) or \
(self.y == point.y and self.x <= point.x <= self.x + self.width) or \
(self.y + self.height == point.y and self.x <= point.x <= self.x + self.width):
return True
else:
return False
class Circle(Point):
def __init__(self, x=0, y=0, radius=0):
super().__init__(x, y)
self.radius = radius
def is_inside(self, point):
distance = math.sqrt((self.x - point.x)**2 + (self.y - point.y)**2)
if distance < self.radius:
return True
elif math.isclose(distance, self.radius):
return True
else:
return False
```
以上代码中,我们定义了一个基类 `Point`,并分别定义了矩形类 `Rectangle` 和圆类 `Circle`,它们都是 `Point` 的派生类。在 `Rectangle` 和 `Circle` 类中,我们分别定义了 `is_inside` 方法,用于判断一个点是否在图形内、在图形的边缘上或在图形外。
在 `Rectangle` 类中,我们使用了矩形的左下角顶点和长宽来定义矩形。在 `is_inside` 方法中,我们先判断点是否在矩形内部,如果在,那么返回 True;如果不在,则判断点是否在矩形的边缘上,如果是,那么也返回 True;否则,返回 False。
在 `Circle` 类中,我们使用圆心和半径来定义圆。在 `is_inside` 方法中,我们计算了点到圆心的距离,如果这个距离小于圆的半径,那么点在圆内部;如果这个距离等于圆的半径,那么点在圆的边缘上;否则,点在圆外部。
你可以使用以下代码来测试上述类的设计是否正确:
```python
p1 = Point(3, 4)
r1 = Rectangle(0, 0, 6, 8)
print(r1.is_inside(p1)) # True
p2 = Point(7, 8)
print(r1.is_inside(p2)) # False
c1 = Circle(0, 0, 5)
print(c1.is_inside(p1)) # True
p3 = Point(5, 0)
print(c1.is_inside(p3)) # True
p4 = Point(6, 0)
print(c1.is_inside(p4)) # False
```
输出结果应该为:
```
True
False
True
True
False
```
希望能对你有所帮助!
阅读全文
相关推荐
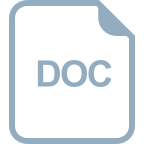
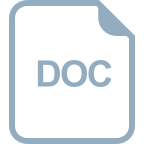
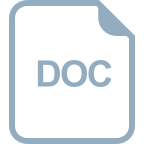
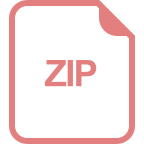
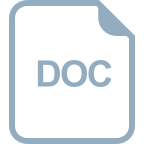
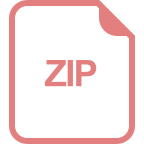










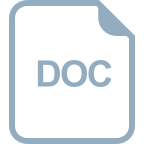
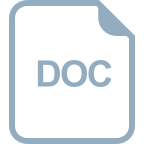