把定义平面直角坐标系上的一个点的类Point作为基类,派生出描述一条直线的类Line
时间: 2024-05-22 22:15:36 浏览: 21
#include <iostream>
using namespace std;
class Point {
protected:
double x;
double y;
public:
Point(double x = 0, double y = 0) {
this->x = x;
this->y = y;
}
double getX() const {
return x;
}
double getY() const {
return y;
}
void setX(double x) {
this->x = x;
}
void setY(double y) {
this->y = y;
}
void setXY(double x, double y) {
this->x = x;
this->y = y;
}
void print() const {
cout << "(" << x << ", " << y << ")";
}
};
class Line : public Point {
private:
double k;
double b;
public:
Line(double k = 0, double b = 0) {
this->k = k;
this->b = b;
}
Line(Point p1, Point p2) {
if (p1.getX() == p2.getX()) {
k = INFINITY;
b = p1.getX();
} else {
k = (p2.getY() - p1.getY()) / (p2.getX() - p1.getX());
b = p1.getY() - k * p1.getX();
}
}
double getK() const {
return k;
}
double getB() const {
return b;
}
void setK(double k) {
this->k = k;
}
void setB(double b) {
this->b = b;
}
void setKB(double k, double b) {
this->k = k;
this->b = b;
}
void print() const {
cout << "y = " << k << "x + " << b;
}
};
int main() {
Point p1(1, 2);
Point p2(3, 4);
Line l1(p1, p2);
l1.print();
return 0;
}
相关推荐
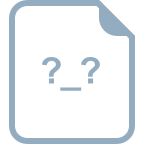
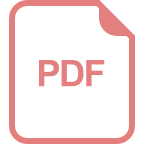
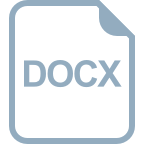














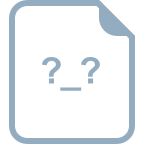