ValueError: The last dimension of the inputs to a Dense layer should be defined. Found None. Full input shape received: (None, None)
时间: 2024-02-20 12:58:53 浏览: 31
这个错误提示表明,在使用 Dense 层时,输入张量的最后一个维度应该是定义好的,但是你提供的输入张量最后一个维度是 None,即未定义。这通常是由于输入数据的形状不正确导致的。
你可以尝试指定输入数据的形状。例如,如果你要输入的是一维向量,你可以使用 `tf.keras.layers.Input` 函数来指定输入张量的形状,如下所示:
```python
import tensorflow as tf
inputs = tf.keras.layers.Input(shape=(10,)) # 假设输入的是长度为 10 的一维向量
x = tf.keras.layers.Dense(32, activation='relu')(inputs)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs=inputs, outputs=outputs)
```
在这个示例中,我们使用 `tf.keras.layers.Input` 函数来指定输入张量的形状为 (batch_size, 10),即输入的是长度为 10 的一维向量。然后,在后续的层中,我们可以使用这个输入张量来定义模型的结构。
你可以根据你的具体情况进行调整。如果你仍然遇到问题,可以提供更多的代码和数据信息,以便我更好地帮助你。
相关问题
ValueError: The last dimension of the inputs to `Dense` should be defined. Found `None`.
这个错误通常是由于在使用 Keras 的 `Dense` 层时没有指定输入张量的最后一维大小所引起的。
在使用 `Dense` 层之前,您需要确保输入张量的最后一维大小已知。您可以使用 `Input` 层来指定输入张量的形状,如下所示:
```python
import tensorflow as tf
from tensorflow.keras.layers import Dense, Input
# 定义输入张量的形状
inputs = Input(shape=(10,))
# 使用 Dense 层时,指定输入张量的最后一维大小
x = Dense(32)(inputs)
# 创建模型
model = tf.keras.Model(inputs=inputs, outputs=x)
```
在上面的代码中,我们使用 `Input` 层来定义输入张量的形状为 `(10,)`。接下来,在使用 `Dense` 层时,我们将输入张量的最后一维大小设置为 `32`,即 `x = Dense(32)(inputs)`。这样就可以避免上述错误的出现。
如何解决这个错误ValueError: The last dimension of the inputs to `Dense` should be defined. Found `None`.
这个错误通常是由于在定义 `Dense` 层时没有指定输入的最后一个维度大小引起的。解决方法是在定义 `Dense` 层时指定输入张量的最后一个维度大小,或者在前面的层中指定输出张量的最后一个维度大小。
例如,如果你想定义一个输入形状为 `(batch_size, input_dim)`,输出形状为 `(batch_size, output_dim)` 的 `Dense` 层,可以这样写:
```python
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model
input_tensor = Input(shape=(input_dim,))
output_tensor = Dense(output_dim)(input_tensor)
model = Model(inputs=input_tensor, outputs=output_tensor)
```
其中,`input_dim` 和 `output_dim` 分别为输入和输出张量的最后一个维度大小,`input_tensor` 和 `output_tensor` 分别为输入和输出张量。
相关推荐
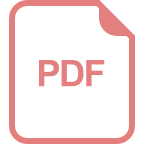
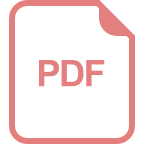
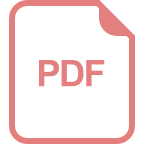









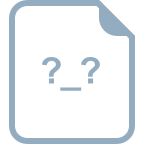
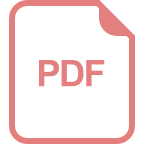
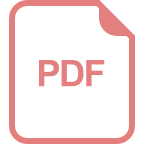
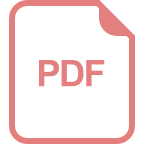