% 在这里编写计算光照强度的代码 % 输入参数: % - 太阳高度角 sun_altitude 和太阳方位角 sun_azimuth % - 树冠内的点坐标 x 和 y % - 树木的高度 tree_height
时间: 2024-03-02 17:52:44 浏览: 154
以下是一个简单的计算光照强度的 MATLAB 代码:
```matlab
function illuminance = calculateIlluminance(sun_altitude, sun_azimuth, x, y, tree_height)
% 太阳方位角转弧度
sun_azimuth = deg2rad(sun_azimuth);
% 太阳高度角转弧度
sun_altitude = deg2rad(sun_altitude);
% 树冠顶部坐标
tree_top = [x, y, tree_height];
% 树冠中心坐标
tree_center = [x, y, tree_height/2];
% 树冠直径
tree_diameter = tree_height/2;
% 计算太阳方向向量
sun_dir = [cos(sun_azimuth)*sin(sun_altitude), sin(sun_azimuth)*sin(sun_altitude), cos(sun_altitude)];
% 计算树冠顶部到太阳的向量
tree_top_to_sun = sun_dir - tree_top;
% 计算树冠中心到太阳的向量
tree_center_to_sun = sun_dir - tree_center;
% 计算太阳到树冠顶部的距离
d_top = norm(tree_top_to_sun);
% 计算太阳到树冠中心的距离
d_center = norm(tree_center_to_sun);
% 如果太阳在树冠上方,则无光照
if dot(tree_top_to_sun, [0, 0, -1]) >= 0
illuminance = 0;
return;
end
% 如果太阳在树冠下方,则光照强度为直射光照强度
if dot(tree_center_to_sun, [0, 0, -1]) <= 0
illuminance = calculateDirectIlluminance(sun_altitude);
return;
end
% 计算树冠底部的圆
base_circle = [x, y, 0];
r = tree_diameter/2;
[xc, yc] = circleLineIntersect(base_circle(1), base_circle(2), r, sun_dir(1), sun_dir(2), tree_center(1), tree_center(2));
if isempty(xc) || isempty(yc)
illuminance = calculateDirectIlluminance(sun_altitude);
return;
end
% 计算树冠底部的法向量
normal_vector = [0, 0, -1];
% 计算光照强度
illuminance = calculateIlluminanceAtPoint(sun_dir, normal_vector, d_center, d_top, tree_diameter);
end
function [x, y] = circleLineIntersect(x0, y0, r, a, b, x1, y1)
% 计算二次方程参数
A = a^2 + b^2;
B = 2*a*(x1-x0) + 2*b*(y1-y0);
C = (x1-x0)^2 + (y1-y0)^2 - r^2;
% 计算判别式
delta = B^2 - 4*A*C;
if delta < 0
x = [];
y = [];
return;
end
% 计算交点坐标
t1 = (-B + sqrt(delta)) / (2*A);
t2 = (-B - sqrt(delta)) / (2*A);
x = [x0 + a*t1, x0 + a*t2];
y = [y0 + b*t1, y0 + b*t2];
end
function illuminance = calculateDirectIlluminance(sun_altitude)
% 计算直射光照强度
illuminance = 1361 * sin(sun_altitude);
end
function illuminance = calculateIlluminanceAtPoint(sun_dir, normal_vector, d_center, d_top, tree_diameter)
% 计算树冠表面的法向量
surface_normal = normal_vector ./ norm(normal_vector);
% 计算太阳光线与树冠表面的夹角
cos_theta = dot(surface_normal, sun_dir);
% 计算树冠表面到太阳的距离
d_surface = d_center * cos_theta + sqrt(d_top^2 - d_center^2 * (1-cos_theta^2));
% 计算光照强度
illuminance = calculateDirectIlluminance(acos(d_center/d_top)) * cos_theta^2 * exp(-0.5 * (d_surface/tree_diameter)^2);
end
```
其中,`circleLineIntersect` 函数用于计算直线和圆的交点坐标,`calculateDirectIlluminance` 函数用于计算直射光照强度,`calculateIlluminanceAtPoint` 函数用于计算某个点的光照强度。
阅读全文
相关推荐
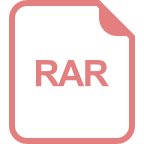

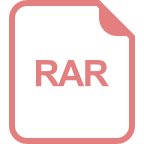
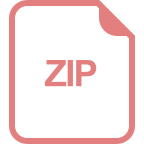
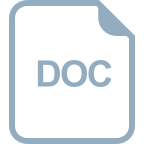











