代码补充b)图像对B1、B2 img1 = np.zeros((100, 100)) img2 = np.zeros((100, 100)) img1[30:70, 30:70] = 1 img2[20:40, 30:70] = 1 img2[60:80, 30:70] = 1 plt.subplot(121) plt.imshow(img1) plt.axis('off') plt.subplot(122) plt.imshow(img2) plt.axis('off') plt.show()
时间: 2023-12-10 13:37:41 浏览: 34
好的,这里是代码补充,完整代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
# 图像 B1
img1 = np.zeros((100, 100))
img1[30:70, 30:70] = 1
# 图像 B2
img2 = np.zeros((100, 100))
img2[20:40, 30:70] = 1
img2[60:80, 30:70] = 1
# 显示图像
plt.subplot(121)
plt.imshow(img1)
plt.axis('off')
plt.subplot(122)
plt.imshow(img2)
plt.axis('off')
plt.show()
```
其中,`np.zeros((100, 100))` 创建了一个大小为 $100 \times 100$ 的全黑图像,然后通过对数组的赋值操作来绘制出需要的图案。`plt.subplot(121)` 和 `plt.subplot(122)` 将整个画布分成两个区域,分别显示两张图像。`plt.imshow()` 用于显示图像,`plt.axis('off')` 用于隐藏坐标轴。最后,`plt.show()` 显示出整个画布。
相关问题
from tkinter import * import cv2 import numpy as np from PIL import ImageGrab from tensorflow.keras.models import load_model from temp import * model = load_model('mnist.h5') image_folder = "img/" root = Tk() root.resizable(0, 0) root.title("HDR") lastx, lasty = None, None image_number = 0 cv = Canvas(root, width=1200, height=480, bg='white') cv.grid(row=0, column=0, pady=2, sticky=W, columnspan=2) def clear_widget(): global cv cv.delete('all') def draw_lines(event): global lastx, lasty x, y = event.x, event.y cv.create_line((lastx, lasty, x, y), width=8, fill='black', capstyle=ROUND, smooth=True, splinesteps=12) lastx, lasty = x, y def activate_event(event): global lastx, lasty cv.bind('<B1-Motion>', draw_lines) lastx, lasty = event.x, event.y cv.bind('<Button-1>', activate_event) def Recognize_Digit(): global image_number filename = f'img_{image_number}.png' root.update() widget = cv x = root.winfo_rootx() + widget.winfo_rootx() y = root.winfo_rooty() + widget.winfo_rooty() x1 = x + widget.winfo_width() y1 = y + widget.winfo_height() print(x, y, x1, y1) # get image and save ImageGrab.grab().crop((x, y, x1, y1)).save(image_folder + filename) image = cv2.imread(image_folder + filename, cv2.IMREAD_COLOR) gray = cv2.cvtColor(image.copy(), cv2.COLOR_BGR2GRAY) ret, th = cv2.threshold( gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU) # contours = cv2.findContours( # th, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[0] Position = findContours(th) for m in range(len(Position)): # make a rectangle box around each curve cv2.rectangle(th, (Position[m][0], Position[m][1]), ( Position[m][2], Position[m][3]), (255, 0, 0), 1) # Cropping out the digit from the image corresponding to the current contours in the for loop digit = th[Position[m][1]:Position[m] [3], Position[m][0]:Position[m][2]] # Resizing that digit to (18, 18) resized_digit = cv2.resize(digit, (18, 18)) # Padding the digit with 5 pixels of black color (zeros) in each side to finally produce the image of (28, 28) padded_digit = np.pad(resized_digit, ((5, 5), (5, 5)), "constant", constant_values=0) digit = padded_digit.reshape(1, 28, 28, 1) digit = digit / 255.0 pred = model.predict([digit])[0] final_pred = np.argmax(pred) data = str(final_pred) + ' ' + str(int(max(pred) * 100)) + '%' print(data) font = cv2.FONT_HERSHEY_SIMPLEX fontScale = 0.5 color = (255, 0, 0) thickness = 1 cv2.putText(th, data, (Position[m][0], Position[m][1] - 5), font, fontScale, color, thickness) cv2.imshow('image', th) cv2.waitKey(0) cv2.destroyAllWindows() btn_save = Button(text='Recognize Digit', command=Recognize_Digit) btn_save.grid(row=2, column=0, pady=1, padx=1) button_clear = Button(text='Clear Widget', command=clear_widget) button_clear.grid(row=2, column=1, pady=1, padx=1) root.mainloop()
这段代码是一个Python程序,用于画图并识别手写数字。它调用了许多库,如tkinter、cv2、numpy、PIL和tensorflow。程序创建了一个图形用户界面,包括一个白色的画布和一个按钮。用户在画布上绘制数字后,可以点击按钮来触发识别数字的功能。程序会将绘制的数字保存为图片文件,并加载训练好的模型(mnist.h5)来识别数字。程序最后打印出保存的图片文件的坐标信息。
自己写一个类似cv2.addWeighted的函数实现图像融合, 不要用cv2.addWeighted
好的,我可以给你提供一种实现图像融合的方法。
方法如下:
1. 首先,读取两张待融合的图片。
2. 然后,将两张图片进行缩放,使得它们的大小相同。
3. 接着,创建一个新的空白图片,大小和融合后的图片大小相同。
4. 对于新的空白图片中的每个像素,计算其RGB值,方法如下:
1. 对于第一张图片中的像素,计算其RGB值的平均值。
2. 对于第二张图片中的像素,计算其RGB值的平均值。
3. 将两个平均值按照一定的比例进行加权求和,得到新的RGB值。
5. 最后,将计算得到的RGB值填充到新的空白图片中,生成融合后的图片。
下面是实现代码:
```python
import numpy as np
import cv2
# 定义图像融合函数
def my_addWeighted(img1, img2, alpha, beta, gamma):
# 缩放两张图片大小
img1 = cv2.resize(img1, (img2.shape[1], img2.shape[0]))
# 创建新的空白图片
img_blend = np.zeros_like(img1)
# 计算融合后的每个像素的RGB值
for i in range(img1.shape[0]):
for j in range(img1.shape[1]):
b1, g1, r1 = img1[i, j]
b2, g2, r2 = img2[i, j]
b_new = alpha * b1 + beta * b2 + gamma
g_new = alpha * g1 + beta * g2 + gamma
r_new = alpha * r1 + beta * r2 + gamma
img_blend[i, j] = [b_new, g_new, r_new]
return img_blend
# 读取两张待融合的图片
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 调用my_addWeighted函数进行图像融合
img_blend = my_addWeighted(img1, img2, 0.5, 0.5, 0)
# 显示融合后的图片
cv2.imshow('img_blend', img_blend)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们使用了一个双重循环来遍历融合后的每个像素,并计算其RGB值。这种方法虽然比较慢,但实现起来比较简单,容易理解。如果你需要更快的图像融合方法,可以使用numpy的矩阵运算来实现。
相关推荐
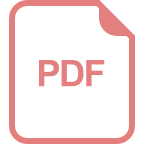
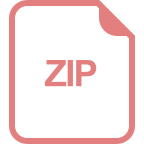










