选修某课程的学生有5人,学生的信息包括学号、姓名和成绩,按成绩高低对学生排序,输出排序后的学生信息。从键盘输入一学生姓名,查出其成绩和成绩等级。(用指向指针的 指针处理学生的信息交换)
时间: 2024-01-01 11:06:13 浏览: 94
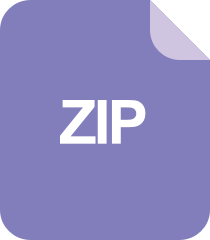
接收从键盘输入某班学生的学号、姓名、成绩,对学 生的成绩进行排序.zip

以下是一个示例代码,实现了上述功能:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Student {
string id;
string name;
double score;
};
void sortStudents(Student** students, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if ((*students[i]).score < (*students[j]).score) {
Student* temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
int main() {
Student* students[5];
students[0] = new Student{"001", "Alice", 85.5};
students[1] = new Student{"002", "Bob", 92.0};
students[2] = new Student{"003", "Charlie", 78.5};
students[3] = new Student{"004", "David", 88.5};
students[4] = new Student{"005", "Eve", 95.0};
sortStudents(students, 5);
for (int i = 0; i < 5; i++) {
cout << (*students[i]).id << " " << (*students[i]).name << " " << (*students[i]).score << endl;
}
string name;
cout << "Please enter a student name:" << endl;
cin >> name;
double score = -1;
char level;
for (int i = 0; i < 5; i++) {
if ((*students[i]).name == name) {
score = (*students[i]).score;
if (score >= 90) {
level = 'A';
} else if (score >= 80) {
level = 'B';
} else if (score >= 70) {
level = 'C';
} else if (score >= 60) {
level = 'D';
} else {
level = 'E';
}
break;
}
}
if (score < 0) {
cout << "No such student found." << endl;
} else {
cout << "Score: " << score << ", Level: " << level << endl;
}
for (int i = 0; i < 5; i++) {
delete students[i];
}
return 0;
}
```
该程序首先定义了一个 `Student` 结构体,包含了学生的三个属性。然后定义了一个 `sortStudents` 函数,用于将学生按照成绩从高到低排序。在 `main` 函数中,我们创建了一个包含5个学生的数组,并调用 `sortStudents` 函数进行排序。然后通过循环输出排好序的学生信息。
接下来,程序从标准输入读入一个学生姓名,并在数组中查找该学生的信息。如果找到该学生,就根据其成绩确定其成绩等级,并输出成绩和等级。最后释放内存,结束程序。
值得注意的是,在 `sortStudents` 函数中,我们使用了指向指针的指针来交换学生信息。这是因为我们需要修改指针数组中的元素,而直接使用指针变量则无法实现。
阅读全文
相关推荐
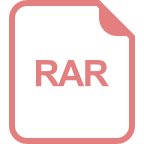
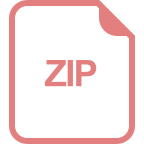






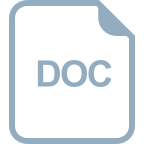
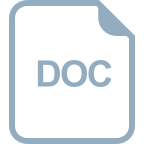
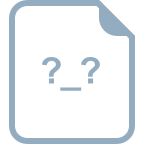
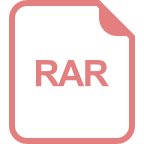
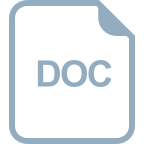
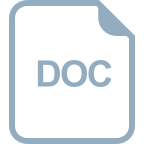
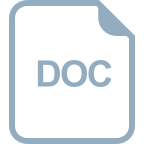
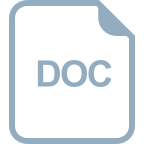