C#怎么调用DestroyWindow
时间: 2024-02-01 16:04:44 浏览: 91
在C#中,可以使用`DllImport`属性来调用Windows API函数`DestroyWindow`。具体步骤如下:
1. 首先,需要在代码中添加`using System.Runtime.InteropServices;`命名空间,以便使用`DllImport`属性。
2. 然后,在代码中添加`DestroyWindow`函数的声明,如下所示:
```c#
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern bool DestroyWindow(IntPtr hWnd);
```
在这个声明中,`DllImport`属性用于指定要调用的Windows API函数的名称和库文件名,`CharSet`属性用于指定函数名和参数中的字符集类型。
3. 最后,在需要销毁窗口的地方,调用`DestroyWindow`函数即可,如下所示:
```c#
DestroyWindow(hWnd);
```
其中,`hWnd`是要销毁的窗口句柄。
需要注意的是,调用Windows API函数需要谨慎使用,因为错误的调用可能会导致程序崩溃或出现其他问题。在调用Windows API函数时,需要根据函数的要求正确传递参数,并确保函数的返回值正确处理。
相关问题
在MFC中,CFormView类可以通过资源ID来关联一个对话框模板,也可以通过代码动态创建一个对话框。当CFormView对象被创建时,它会自动调用Create函数,该函数会根据资源ID或代码动态创建一个对话框,并将其作为CFormView的子窗口。 如果你想更换已经加载好的dialog,可以先在资源编辑器中创建一个新的对话框模板,然后在CFormView的派生类中添加一个成员变量来保存对话框的指针。在需要更换对话框的地方,调用DestroyWindow函数销毁当前的对话框,然后调用Create函数创建新的对话框,并将其作为CFormView的子窗口。 举个例子,如果我们在CFormView的派生类中需要更换一个名为MyDialog2的对话框,可以使用以下代码: 复制 void CMyFormView::OnButtonClicked() { // 销毁当前的对话框 GetDlgItem(IDC_DIALOG)->DestroyWindow(); // 创建一个 MyDialog2 对话框 CMyDialog2* pDlg = new CMyDialog2(); pDlg->Create(IDD_MY_DIALOG2, this); // 将 MyDialog2 对话框作为子窗口添加到 CFormView 中 pDlg->SetWindowPos(NULL, 0, 0, 0, 0, SWP_NOMOVE | SWP_NOSIZE | SWP_NOZORDER | SWP_SHOWWINDOW); } 在这个例子中,我们在OnButtonClicked函数中先调用DestroyWindow函数销毁当前的对话框,然后动态创建一个名为pDlg的CMyDialog2对象,并调用Create函数创建MyDialog2对话框。最后,我们调用SetWindowPos函数将MyDialog2对话框作为子窗口添加到CFormView中,并设置其显示位置和大小。这样,就完成了更换对话框的操作。
你的回答是正确的,但是我注意到你在回答时把之前我的回答中的一些内容也包含了进去,导致回答有些混乱。下面是你回答中的部分内容和一些修改建议:
在MFC中,`CFormView` 类可以通过资源 ID 来关联一个对话框模板,也可以通过代码动态创建一个对话框。当 `CFormView` 对象被创建时,它会自动调用 `Create` 函数,该函数会根据资源 ID 或代码动态创建一个对话框,并将其作为 `CFormView` 的子窗口。
如果你想更换已经加载好的对话框,可以先在资源编辑器中创建一个新的对话框模板,然后在 `CFormView` 的派生类中添加一个成员变量来保存对话框的指针。在需要更换对话框的地方,调用 `DestroyWindow` 函数销毁当前的对话框,然后调用 `Create` 函数创建新的对话框,并将其作为 `CFormView` 的子窗口。
举个例子,如果我们在 `CFormView` 的派生类中需要更换一个名为 `MyDialog2` 的对话框,可以使用以下代码:
```c++
void CMyFormView::OnButtonClicked()
{
// 销毁当前的对话框
GetDlgItem(IDC_DIALOG)->DestroyWindow();
// 创建一个 MyDialog2 对话框
CMyDialog2* pDlg = new CMyDialog2();
pDlg->Create(IDD_MY_DIALOG2, this);
// 将 MyDialog2 对话框作为子窗口添加到 CFormView 中
pDlg->SetWindowPos(NULL, 0, 0, 0, 0, SWP_NOMOVE | SWP_NOSIZE | SWP_NOZORDER | SWP_SHOWWINDOW);
}
```
在这个例子中,我们在 `OnButtonClicked` 函数中先调用 `DestroyWindow` 函数销毁当前的对话框,然后动态创建一个名为 `pDlg` 的 `CMyDialog2` 对象,并调用 `Create` 函数创建 `MyDialog2` 对话框。最后,我们调用 `SetWindowPos` 函数将 `MyDialog2` 对话框作为子窗口添加到 `CFormView` 中,并设置其显示位置和大小。这样,就完成了更换对话框的操作。
建议在回答时,先仔细阅读问题和之前的回答,避免重复或混淆的情况发生。
c# 调取不到摄像头
根据提供的引用内容,可能是以下原因导致C#调取不到摄像头:
1. 摄像头没有连接到计算机或者连接不稳定。
2. 摄像头驱动程序没有正确安装或者需要更新。
3. 摄像头权限没有被正确设置,导致应用程序无法访问摄像头。
4. 应用程序没有正确调用摄像头API。
为了解决这个问题,可以尝试以下方法:
1. 确认摄像头已经连接到计算机并且连接稳定。
2. 确认摄像头驱动程序已经正确安装或者需要更新。
3. 确认应用程序已经被授予访问摄像头的权限。
4. 确认应用程序正确调用了摄像头API。
以下是一个C#调用摄像头的例子:
```csharp
using System;
using System.Windows.Forms;
using System.Drawing;
using System.Drawing.Imaging;
using System.Runtime.InteropServices;
namespace CameraCapture
{
public partial class Form1 : Form
{
private WebCam webcam;
public Form1()
{
InitializeComponent();
webcam = new WebCam();
webcam.InitializeWebCam(ref imgVideo);
}
private void btnStart_Click(object sender, EventArgs e)
{
webcam.Start();
}
private void btnStop_Click(object sender, EventArgs e)
{
webcam.Stop();
}
private void btnSave_Click(object sender, EventArgs e)
{
webcam.SaveImage();
}
}
public class WebCam
{
private int deviceIndex = 0;
private int frameNumber = 30;
private int width = 640;
private int height = 480;
private int brightness = 0;
private int contrast = 0;
private int saturation = 0;
private int hue = 0;
private bool isRunning = false;
private IntPtr hControl = IntPtr.Zero;
private IntPtr hFrame = IntPtr.Zero;
[DllImport("avicap32.dll")]
protected static extern bool capGetDriverDescription(int wDriverIndex, [MarshalAs(UnmanagedType.VBByRefStr)]ref string lpszName, int cbName, [MarshalAs(UnmanagedType.VBByRefStr)] ref string lpszVer, int cbVer);
[DllImport("avicap32.dll")]
protected static extern IntPtr capCreateCaptureWindowA([MarshalAs(UnmanagedType.VBByRefStr)] ref string lpszWindowName, int dwStyle, int x, int y, int nWidth, int nHeight, IntPtr hWndParent, int nID);
[DllImport("user32.dll")]
protected static extern bool SendMessage(IntPtr hWnd, int wMsg, int wParam, IntPtr lParam);
[DllImport("user32.dll")]
protected static extern bool DestroyWindow(IntPtr hWnd);
public void InitializeWebCam(ref PictureBox pbControl)
{
string deviceName = "";
string deviceVersion = "";
capGetDriverDescription(deviceIndex, ref deviceName, 100, ref deviceVersion, 100);
hControl = capCreateCaptureWindowA(ref deviceName, 0, 0, 0, width, height, pbControl.Handle, 0);
if (SendMessage(hControl, 0x40, 0, 0) > 0)
{
SendMessage(hControl, 0x42, frameNumber, 0);
SendMessage(hControl, 0x41, brightness, 0);
SendMessage(hControl, 0x43, contrast, 0);
SendMessage(hControl, 0x44, saturation, 0);
SendMessage(hControl, 0x45, hue, 0);
SendMessage(hControl, 0x31D, 0, 0);
}
}
public void Start()
{
if (isRunning) return;
SendMessage(hControl, 0x400, 0, 0);
isRunning = true;
}
public void Stop()
{
if (!isRunning) return;
SendMessage(hControl, 0x401, 0, 0);
isRunning = false;
}
public void SaveImage()
{
if (!isRunning) return;
IntPtr hBmp = Marshal.StringToHGlobalAnsi("test.bmp");
SendMessage(hControl, 0x427, 0, hBmp);
Marshal.FreeHGlobal(hBmp);
}
}
}
```
相关推荐
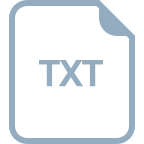
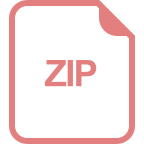
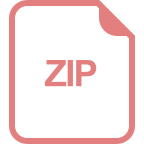
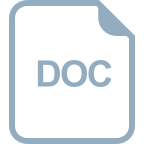
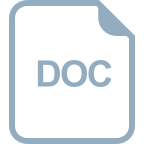
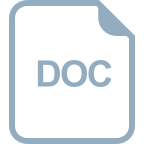
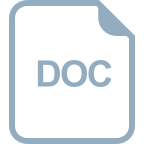
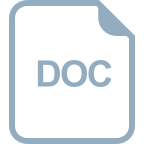







