c# 读取实时内存视频流 播放
时间: 2023-07-27 10:28:15 浏览: 66
要读取实时内存视频流并播放,您可以使用FFmpeg库。FFmpeg是一个跨平台的开源库,可以用于处理音频和视频文件,并且它还支持实时视频流处理。
以下是一个简单的C#代码示例,可以使用FFmpeg库来读取实时内存视频流并播放:
```csharp
using System;
using FFmpeg.AutoGen;
namespace VideoPlayer
{
class Program
{
static void Main(string[] args)
{
// 初始化FFmpeg库
ffmpeg.av_register_all();
ffmpeg.avcodec_register_all();
// 创建AVFormatContext对象
AVFormatContext* pFormatContext = null;
ffmpeg.avformat_open_input(&pFormatContext, "your_memory_video_stream_address", null, null);
// 查找视频流
ffmpeg.avformat_find_stream_info(pFormatContext, null);
int videoStreamIndex = ffmpeg.av_find_best_stream(pFormatContext, AVMediaType.AVMEDIA_TYPE_VIDEO, -1, -1, null, 0);
// 获取视频解码器
AVCodecParameters* pCodecParameters = pFormatContext->streams[videoStreamIndex]->codecpar;
AVCodec* pCodec = ffmpeg.avcodec_find_decoder(pCodecParameters->codec_id);
AVCodecContext* pCodecContext = ffmpeg.avcodec_alloc_context3(pCodec);
ffmpeg.avcodec_parameters_to_context(pCodecContext, pCodecParameters);
ffmpeg.avcodec_open2(pCodecContext, pCodec, null);
// 创建AVFrame对象
AVFrame* pFrame = ffmpeg.av_frame_alloc();
AVPacket packet = new AVPacket();
// 创建Sdl窗口
SDL.SDL_Init(SDL.SDL_INIT_VIDEO);
SDL.SDL_Window* pWindow = SDL.SDL_CreateWindow("VideoPlayer", SDL.SDL_WINDOWPOS_CENTERED, SDL.SDL_WINDOWPOS_CENTERED, pCodecContext->width, pCodecContext->height, SDL.SDL_WindowFlags.SDL_WINDOW_SHOWN);
SDL.SDL_Renderer* pRenderer = SDL.SDL_CreateRenderer(pWindow, -1, SDL.SDL_RendererFlags.SDL_RENDERER_ACCELERATED);
// 播放视频
while (ffmpeg.av_read_frame(pFormatContext, &packet) >= 0)
{
if (packet.stream_index == videoStreamIndex)
{
ffmpeg.avcodec_send_packet(pCodecContext, &packet);
while (ffmpeg.avcodec_receive_frame(pCodecContext, pFrame) >= 0)
{
SDL.SDL_Texture* pTexture = SDL.SDL_CreateTexture(pRenderer, SDL.SDL_PIXELFORMAT_YV12, (int)SDL.SDL_TextureAccess.SDL_TEXTUREACCESS_STREAMING, pCodecContext->width, pCodecContext->height);
SDL.SDL_UpdateYUVTexture(pTexture, null, pFrame->data[0], pFrame->linesize[0], pFrame->data[1], pFrame->linesize[1], pFrame->data[2], pFrame->linesize[2]);
SDL.SDL_RenderClear(pRenderer);
SDL.SDL_RenderCopy(pRenderer, pTexture, null, null);
SDL.SDL_RenderPresent(pRenderer);
SDL.SDL_DestroyTexture(pTexture);
}
}
ffmpeg.av_packet_unref(&packet);
}
// 释放资源
SDL.SDL_DestroyRenderer(pRenderer);
SDL.SDL_DestroyWindow(pWindow);
ffmpeg.av_frame_free(&pFrame);
ffmpeg.avcodec_close(pCodecContext);
ffmpeg.avformat_close_input(&pFormatContext);
SDL.SDL_Quit();
}
}
}
```
在这个示例中,我们使用SDL库来创建窗口并播放视频。您可以通过NuGet包管理器来安装SDL2库。请注意,这只是一个简单的示例,您需要根据实际情况进行修改和适应。
相关推荐
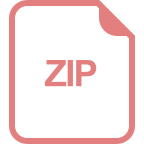
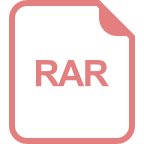














