【问题描述】 从给定列表中找出只出现一次的数,如:给出[1,2,2,3,1,4,3],返回4。 【样例输入】 1 2 2 3 1 4 3 【样例输出】 4
时间: 2024-05-16 20:16:35 浏览: 59
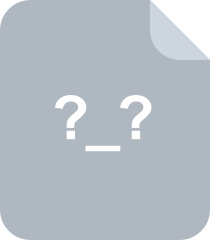
数组中唯一只出现一次的数字.md
以下是一个 Python 的实现:
```python
from collections import Counter
def find_unique(nums):
counts = Counter(nums)
for num, count in counts.items():
if count == 1:
return num
return None
nums = list(map(int, input().split()))
print(find_unique(nums))
```
我们使用了 Python 内置的 `collections` 模块中的 `Counter` 类来统计每个数字出现的次数,然后遍历这个计数器,找到出现次数为 1 的数字即可。注意,如果没有只出现一次的数字,函数会返回 `None`。
阅读全文
相关推荐
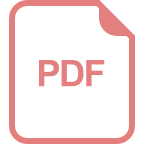














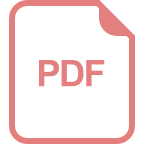