java在student类、teacher类和people类的基础上,将student类和teacher类改成people类的子类。并尝试将成员变量或方法设置成不同的访问权限(public、private、protected、友好)。
时间: 2024-05-03 22:17:57 浏览: 163
好的,我来给您演示一下。
首先,我们先定义一个 `People` 类,作为 `Student` 和 `Teacher` 的父类。
```java
public class People {
private String name;
protected int age;
public String gender;
public People(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
public void introduce() {
System.out.println("My name is " + name + ", I'm " + age + " years old. I'm a " + gender + ".");
}
}
```
在 `People` 类中,我们定义了三个成员变量 `name`、`age` 和 `gender`,其中 `name` 是私有的,`age` 是受保护的,`gender` 是公有的。此外,我们还定义了一个 `introduce()` 方法用于介绍自己。
接下来,我们定义 `Student` 和 `Teacher` 类,它们都是 `People` 类的子类。
```java
public class Student extends People {
private String school;
public Student(String name, int age, String gender, String school) {
super(name, age, gender);
this.school = school;
}
public void study() {
System.out.println("I'm a student from " + school + ", and I'm studying hard.");
}
}
public class Teacher extends People {
protected String course;
public Teacher(String name, int age, String gender, String course) {
super(name, age, gender);
this.course = course;
}
protected void teach() {
System.out.println("I'm a teacher of " + course + ", and I'm teaching now.");
}
}
```
在 `Student` 类中,我们定义了一个新的成员变量 `school`,它是私有的。此外,我们还定义了一个 `study()` 方法用于学习。
在 `Teacher` 类中,我们重新定义了 `course` 成员变量,它是受保护的。此外,我们还定义了一个 `teach()` 方法用于教学。
最后,我们来测试一下这些类的访问权限:
```java
public class Main {
public static void main(String[] args) {
People p = new People("Tom", 20, "male");
p.introduce();
// 输出:My name is Tom, I'm 20 years old. I'm a male.
Student s = new Student("Alice", 18, "female", "ABC School");
s.introduce();
// 输出:My name is Alice, I'm 18 years old. I'm a female.
s.study();
// 输出:I'm a student from ABC School, and I'm studying hard.
System.out.println(s.name);
// 编译错误,name 是私有的,无法访问
Teacher t = new Teacher("Bob", 30, "male", "Math");
t.introduce();
// 输出:My name is Bob, I'm 30 years old. I'm a male.
t.teach();
// 输出:I'm a teacher of Math, and I'm teaching now.
System.out.println(t.gender);
// 输出:male,gender 是公有的,可以访问
}
}
```
在这段代码中,我们创建了一个 `People` 对象 `p`、一个 `Student` 对象 `s` 和一个 `Teacher` 对象 `t`。我们调用了它们的不同方法,并访问了它们的成员变量。
可以看到,我们成功地定义了不同访问权限的成员变量和方法,并且通过继承和多态性实现了不同类之间的相互调用。
阅读全文
相关推荐
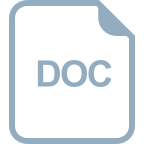
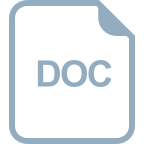
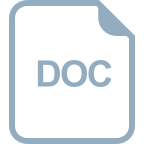


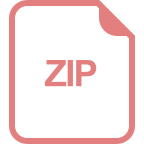
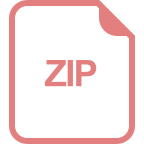
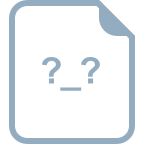










