给我写一个有敌人的python吃豆人小游戏
时间: 2024-05-05 15:21:27 浏览: 16
好的,下面是一个简单的Python吃豆人小游戏,包含敌人和豆子。游戏规则是吃掉所有豆子,避免被敌人撞到。游戏使用Python的pygame库实现。
首先,需要安装pygame库,可以使用以下命令进行安装:
```
pip install pygame
```
然后,创建一个名为“pacman.py”的Python文件,并将以下代码复制到文件中:
```python
import pygame
import random
# 游戏初始化
pygame.init()
# 游戏窗口大小
WIDTH = 640
HEIGHT = 480
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
RED = (255, 0, 0)
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
# 游戏时钟
clock = pygame.time.Clock()
# 加载图片
pacman_img = pygame.image.load('pacman.png')
enemy_img = pygame.image.load('enemy.png')
dot_img = pygame.image.load('dot.png')
# 游戏对象类
class GameObject:
def __init__(self, x, y, image):
self.x = x
self.y = y
self.image = image
def draw(self):
screen.blit(self.image, (self.x, self.y))
# 玩家类
class Player(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 5
def update(self, dx, dy):
self.x += dx * self.speed
self.y += dy * self.speed
def collide(self, other):
return self.x < other.x + 32 and \
self.x + 32 > other.x and \
self.y < other.y + 32 and \
self.y + 32 > other.y
# 敌人类
class Enemy(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 3
self.dx = 1
self.dy = 0
def update(self):
self.x += self.dx * self.speed
self.y += self.dy * self.speed
def change_direction(self):
self.dx = random.randint(-1, 1)
self.dy = random.randint(-1, 1)
# 豆子类
class Dot(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
def collide(self, other):
return self.x < other.x + 32 and \
self.x + 32 > other.x and \
self.y < other.y + 32 and \
self.y + 32 > other.y
# 创建玩家对象
player = Player(320, 240, pacman_img)
# 创建敌人对象
enemies = []
for i in range(5):
x = random.randint(0, WIDTH - 32)
y = random.randint(0, HEIGHT - 32)
enemy = Enemy(x, y, enemy_img)
enemies.append(enemy)
# 创建豆子对象
dots = []
for i in range(50):
x = random.randint(0, WIDTH - 32)
y = random.randint(0, HEIGHT - 32)
dot = Dot(x, y, dot_img)
dots.append(dot)
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 处理玩家移动
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player.update(-1, 0)
if keys[pygame.K_RIGHT]:
player.update(1, 0)
if keys[pygame.K_UP]:
player.update(0, -1)
if keys[pygame.K_DOWN]:
player.update(0, 1)
# 处理敌人移动和碰撞
for enemy in enemies:
enemy.update()
if enemy.x < 0 or enemy.x > WIDTH - 32 or \
enemy.y < 0 or enemy.y > HEIGHT - 32:
enemy.change_direction()
if player.collide(enemy):
running = False
# 处理豆子碰撞
for dot in dots:
if player.collide(dot):
dots.remove(dot)
dot.draw()
# 绘制游戏对象
screen.fill(BLACK)
player.draw()
for enemy in enemies:
enemy.draw()
for dot in dots:
dot.draw()
pygame.display.flip()
# 控制游戏帧率
clock.tick(60)
# 退出游戏
pygame.quit()
```
在同一目录下,需要有以下图片文件:pacman.png,enemy.png和dot.png。可以自行搜索并下载这些图片。
运行游戏,使用方向键控制吃豆人移动,避免被敌人撞到,吃掉所有豆子即可胜利。
相关推荐
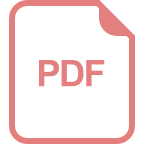
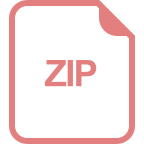














