C++设计一个Point类,包含数据成员x,y,写出构造函数、拷贝构造函数。 再设计一个Rect类(矩形),包含代表左上角顶点的Point类型的数据成员p1,长double len,宽double width及其它必要的数据成员(自己考虑)。写出构造函数、拷贝构造函数,计算矩形面积的函数,计算矩形周长的函数,返回矩形其它顶点的函数(返回类型为Point)。在主函数中创建Rect对象,测试相关功能。
时间: 2023-03-24 18:01:26 浏览: 84
以下是C++中Point和Rect类的代码实现,包括构造函数、拷贝构造函数、析构函数和成员函数。
```cpp
#include <cmath>
class Point {
public:
double x;
double y;
Point(double x_, double y_) : x(x_), y(y_) {}
Point(const Point& p) : x(p.x), y(p.y) {}
~Point() {}
double distance(const Point& p) const {
return std::sqrt(std::pow(x - p.x, 2) + std::pow(y - p.y, 2));
}
};
class Rect {
public:
Point p1;
double len;
double width;
Rect(const Point& p1_, double len_, double width_) : p1(p1_), len(len_), width(width_) {}
Rect(const Rect& r) : p1(r.p1), len(r.len), width(r.width) {}
~Rect() {}
double area() const {
return len * width;
}
double perimeter() const {
return 2 * (len + width);
}
Point upperLeft() const {
return p1;
}
Point upperRight() const {
return Point(p1.x + len, p1.y);
}
Point lowerLeft() const {
return Point(p1.x, p1.y + width);
}
Point lowerRight() const {
return Point(p1.x + len, p1.y + width);
}
};
```
在上面的代码中,Point类包含了两个数据成员x和y,代表点的坐标。它还包含了一个构造函数、拷贝构造函数、析构函数和一个计算点之间距离的成员函数。Rect类包含了一个Point类型的成员变量p1、两个double类型的成员变量len和width,分别表示左上角的点、长度和宽度。它还包含了一个构造函数、拷贝构造函数、析构函数和一些计算面积、周长和各个角点坐标的成员函数。
相关推荐
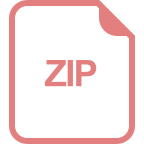
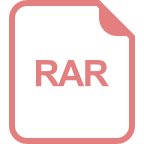














