用C++代码定义一个Point类表示平面上的一个点,再定义一个Rectangle 类表示矩形。Rectangle类中包含Point 类的对象,作为矩形左上角的坐标。要求为Rectangle类创建带参数的构造函数,并在其中初始化Point类的内嵌对象。
时间: 2024-05-15 18:18:39 浏览: 135
以下是用 C 代码定义 Point 类和 Rectangle 类的示例代码:
```c
#include <stdio.h>
// 定义 Point 类
typedef struct {
int x;
int y;
} Point;
// 定义 Rectangle 类
typedef struct {
Point topLeft;
int width;
int height;
} Rectangle;
// 带参数的 Rectangle 类构造函数
Rectangle createRectangle(int x, int y, int width, int height) {
Rectangle rect;
rect.topLeft.x = x;
rect.topLeft.y = y;
rect.width = width;
rect.height = height;
return rect;
}
int main() {
// 创建一个 Rectangle 对象
Rectangle rect = createRectangle(10, 20, 30, 40);
// 打印 Rectangle 对象的属性
printf("Rectangle: (%d, %d) %dx%d\n", rect.topLeft.x, rect.topLeft.y, rect.width, rect.height);
return 0;
}
```
在上述代码中,我们定义了 Point 类和 Rectangle 类,其中 Rectangle 类包含 Point 类的对象作为矩形的左上角坐标。我们还定义了一个带参数的构造函数 createRectangle,用来初始化 Rectangle 类的对象。在 main 函数中,我们创建了一个 Rectangle 对象,并打印出其属性。
阅读全文
相关推荐
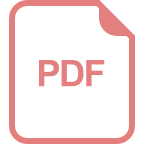
















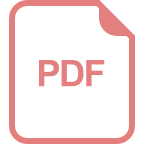