C++定义并实现一个名为Rectangle的矩形类,其属性为矩形的左下角与右上角两个点的坐标,能计算矩形的面积。
时间: 2024-11-17 21:30:43 浏览: 17
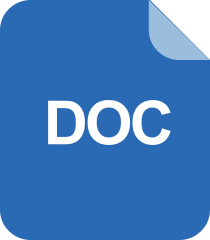
按以下描述和要求建立两个类:基类 Rectangle(矩形类) 和派生类 Cube(正方体)
在C++中,我们可以定义一个名为`Rectangle`的类来表示矩形,它包含两个点(通常是坐标)来描述矩形的边界。这里是一个简单的实现示例:
```cpp
#include <iostream>
#include <cmath>
class Point {
public:
double x;
double y;
Point(double x = 0, double y = 0) : x(x), y(y) {}
};
class Rectangle {
private:
Point topLeft; // 矩形左下角
Point bottomRight; // 矩形右上角
public:
Rectangle(Point topLeft, Point bottomRight)
: topLeft(topLeft), bottomRight(bottomRight) {}
// 计算矩形面积
double getArea() const {
return std::abs(topLeft.x - bottomRight.x) * std::abs(topLeft.y - bottomRight.y);
}
// 输出矩形信息
void print() const {
std::cout << "Top-left corner: (" << topLeft.x << ", " << topLeft.y << ")\n";
std::cout << "Bottom-right corner: (" << bottomRight.x << ", " << bottomRight.y << ")\n";
std::cout << "Area: " << getArea() << "\n";
}
};
int main() {
Point leftBottom(0, 0); // (0, 0)坐标
Point rightUpper(5, 4); // (5, 4)坐标
Rectangle rectangle(leftBottom, rightUpper);
rectangle.print();
return 0;
}
```
在这个例子中,我们首先定义了一个名为`Point`的简单类来存储二维坐标,然后在`Rectangle`类中包含了这两个点作为成员,并提供了一个`getArea`方法来计算面积。`print`方法用于显示矩形的信息。
阅读全文
相关推荐
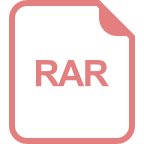
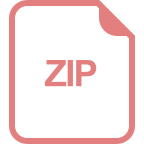















