测试一个名为Rectangle的矩形类,其属性为矩形的左下角与右上角的两点坐标。计算矩形面积。用C++写
时间: 2024-11-13 18:41:22 浏览: 23
在C++中,我们可以创建一个Rectangle类,它包含两个Point类型的成员变量,分别表示矩形的左下角(x1, y1)和右上角(x2, y2),然后定义一个方法来计算并返回矩形的面积。这里是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义一个点类 Point
class Point {
public:
int x, y;
Point(int x = 0, int y = 0): x(x), y(y) {}
};
// 矩形类 Rectangle
class Rectangle {
private:
Point topLeft; // 左下角
Point topRight; // 右上角
public:
// 构造函数设置矩形的左右下角坐标
Rectangle(Point leftBottom, Point rightTop) : topLeft(leftBottom), topRight(rightTop) {}
// 计算并返回矩形面积
int calculateArea() const {
return (topRight.x - topLeft.x) * (topRight.y - topLeft.y);
}
// 打印矩形信息
void print() const {
cout << "Rectangle from (" << topLeft.x << ", " << topLeft.y << ") to (" << topRight.x << ", " << topRight.y << ")" << endl;
cout << "Area: " << calculateArea() << endl;
}
};
int main() {
// 创建矩形实例,并打印信息和面积
Point leftBottom(0, 0); // 点(0,0)
Point rightTop(5, 4); // 点(5,4)
Rectangle rectangle(leftBottom, rightTop);
rectangle.print(); // 输出矩形信息
cout << "Area of the rectangle is: " << rectangle.calculateArea() << endl;
return 0;
}
```
在这个例子中,`calculateArea()`方法用于计算矩形的面积,并在`print()`方法中显示矩形的边界和面积。
阅读全文
相关推荐
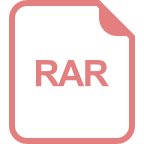
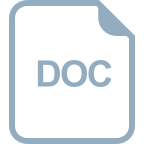
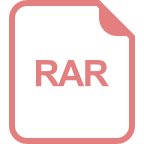
















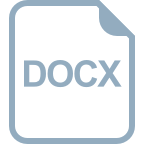