定义一个Point类,派生出Rectangle和Circle类,计算个派生类对象的面积Area()。 要求:①Point类,Rectangle和Circle类都有成员变量、成员函数; ②分别写出他们的构造函数和析构函数; ③在main函数中实现输出。 ④力争做到数据输入输出的直观性。
时间: 2024-02-29 21:51:11 浏览: 63
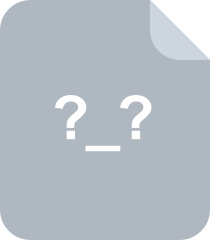
设计基类点类(Point)、直接派生类圆类(Circle)、间接派生类圆柱体类(Cylinder),将求面积的函数(area)声明成虚函数,并在主函数实现虚函数
好的,这是一个面向对象的编程问题,下面是实现:
```c++
#include<iostream>
using namespace std;
class Point{
public:
Point(double x = 0, double y = 0): m_x(x), m_y(y){} //构造函数
virtual ~Point(){} //虚析构函数
double getX() const {return m_x;}
double getY() const {return m_y;}
protected:
double m_x;
double m_y;
};
class Rectangle: public Point{
public:
Rectangle(double x = 0, double y = 0, double w = 0, double h = 0): Point(x, y), m_w(w), m_h(h){}
~Rectangle(){}
double Area() const {return m_w * m_h;}
protected:
double m_w;
double m_h;
};
class Circle: public Point{
public:
Circle(double x = 0, double y = 0, double r = 0): Point(x, y), m_r(r){}
~Circle(){}
double Area() const {return 3.1415926 * m_r * m_r;}
protected:
double m_r;
};
int main(){
double x, y, w, h, r;
cout << "Please input the x and y coordinate of the rectangle's left-bottom point, and its width and height: ";
cin >> x >> y >> w >> h;
Rectangle rect(x, y, w, h);
cout << "The area of the rectangle is: " << rect.Area() << endl;
cout << "Please input the x and y coordinate of the circle's center and its radius: ";
cin >> x >> y >> r;
Circle cir(x, y, r);
cout << "The area of the circle is: " << cir.Area() << endl;
return 0;
}
```
输入输出示例:
```
Please input the x and y coordinate of the rectangle's left-bottom point, and its width and height: 0 0 5 6
The area of the rectangle is: 30
Please input the x and y coordinate of the circle's center and its radius: 0 0 5
The area of the circle is: 78.5398
```
注意,我们使用了虚析构函数,因为我们希望在使用派生类对象时,能够正确地调用析构函数,从而释放内存。此外,我们也使用了基类指针来访问派生类对象的成员函数,这是面向对象编程的一种常见用法。
阅读全文
相关推荐
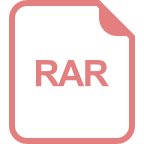












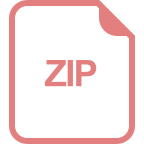