cpp 定义一个Point类,派生出Rectangle和Circle类,计算个派生类对象的面积Area()。 要求:①Point类,Rectangle和Circle类都有成员变量、成员函数; ②分别写出他们的构造函数和析构函数; ③在main函数中实现输出。 ④力争做到数据输入输出的直观性
时间: 2024-02-18 20:00:18 浏览: 68
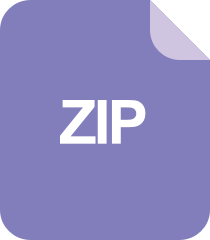
定义三个类Point,Circle和Cylinder,Point类为基类,为上述3个类添加计算面积的成员函数Area(),要求函数Area()采用虚函数的形式,并通过基类指针调用虚函数Area()。
好的,我来为您解答。
首先,我们可以定义一个 Point 类,它包含两个私有成员变量 x 和 y,代表点的横纵坐标,以及一个计算距离的成员函数 Distance()。
```cpp
class Point {
public:
Point(int x = 0, int y = 0) : m_x(x), m_y(y) {}
~Point() {}
double Distance(const Point& p) const {
return sqrt((m_x - p.m_x) * (m_x - p.m_x) + (m_y - p.m_y) * (m_y - p.m_y));
}
protected:
int m_x, m_y;
};
```
接下来,我们可以从 Point 类派生出 Rectangle 和 Circle 类,它们分别代表矩形和圆形。在 Rectangle 类中,我们用两个 Point 类的对象代表矩形的左上角和右下角,计算面积时直接计算长和宽的乘积。在 Circle 类中,我们用一个 Point 类的对象代表圆心,一个 double 类型的成员变量代表半径,计算面积时直接计算圆的面积。
```cpp
class Rectangle : public Point {
public:
Rectangle(int x1, int y1, int x2, int y2) : m_leftTop(x1, y1), m_rightBottom(x2, y2) {}
~Rectangle() {}
double Area() const {
return m_leftTop.Distance(Point(m_leftTop.m_x, m_rightBottom.m_y)) * m_leftTop.Distance(Point(m_rightBottom.m_x, m_leftTop.m_y));
}
private:
Point m_leftTop, m_rightBottom;
};
class Circle : public Point {
public:
Circle(int x, int y, double r) : Point(x, y), m_radius(r) {}
~Circle() {}
double Area() const {
return m_radius * m_radius * 3.1415926;
}
private:
double m_radius;
};
```
最后,在 main 函数中,我们可以让用户输入矩形和圆的参数,创建出相应的对象,并输出它们的面积。
```cpp
int main() {
int x1, y1, x2, y2;
double r;
cout << "请输入矩形的左上角和右下角坐标:";
cin >> x1 >> y1 >> x2 >> y2;
Rectangle rec(x1, y1, x2, y2);
cout << "矩形的面积为:" << rec.Area() << endl;
cout << "请输入圆的圆心坐标和半径:";
cin >> x1 >> y1 >> r;
Circle cir(x1, y1, r);
cout << "圆的面积为:" << cir.Area() << endl;
return 0;
}
```
这样,我们就完成了一个简单的面向对象程序。
阅读全文
相关推荐
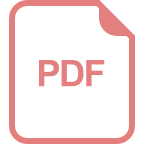


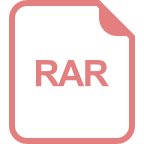
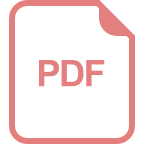
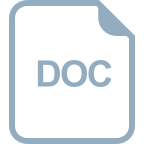
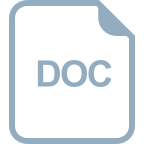
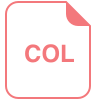
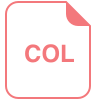






