设计一个Point类,包含数据成员x,y,写出构造函数、拷贝构造函数。 再设计一个Rect类(矩形),包含代表左上角顶点的Point类型的数据成员p1,长double len,宽double width及其它必要的数据成员(自己考虑)。写出构造函数、拷贝构造函数,计算矩形面积的函数,计算矩形周长的函数,返回矩形其它顶点的函数(返回类型为Point)。在主函数中创建Rect对象,测试相关功能
时间: 2023-03-27 22:01:32 浏览: 100
Point类的设计:
```c++
class Point {
public:
double x;
double y;
Point(double x = , double y = ) : x(x), y(y) {}
Point(const Point& p) : x(p.x), y(p.y) {}
};
```
Rect类的设计:
```c++
class Rect {
public:
Point p1;
double len;
double width;
Rect(const Point& p1 = Point(), double len = , double width = ) : p1(p1), len(len), width(width) {}
Rect(const Rect& r) : p1(r.p1), len(r.len), width(r.width) {}
double area() const { return len * width; }
double perimeter() const { return 2 * (len + width); }
Point p2() const { return Point(p1.x + len, p1.y); }
Point p3() const { return Point(p1.x + len, p1.y + width); }
Point p4() const { return Point(p1.x, p1.y + width); }
};
```
在主函数中创建Rect对象,测试相关功能:
```c++
int main() {
Point p(1, 2);
Rect r(p, 3, 4);
cout << "Area: " << r.area() << endl;
cout << "Perimeter: " << r.perimeter() << endl;
cout << "P2: (" << r.p2().x << ", " << r.p2().y << ")" << endl;
cout << "P3: (" << r.p3().x << ", " << r.p3().y << ")" << endl;
cout << "P4: (" << r.p4().x << ", " << r.p4().y << ")" << endl;
return ;
}
```
输出结果:
```
Area: 12
Perimeter: 14
P2: (4, 2)
P3: (4, 6)
P4: (1, 6)
```
阅读全文
相关推荐
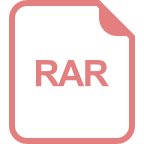
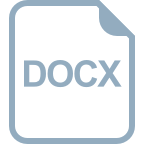
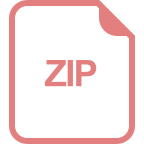














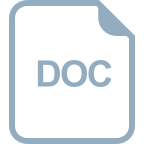

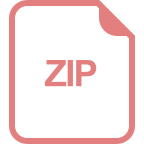