把定义平面直角坐标系上的一个点的类Point作为基类,派生出描述一条直线的类Line,在派生出一个矩形类Rect,要求成员函数能求出两点距离,矩形的周长和面积额,设计一个测试程序,并构造完整的程序
时间: 2024-05-23 18:15:41 浏览: 19
#include <iostream>
#include <cmath>
using namespace std;
class Point {
protected:
double x, y;
public:
Point(double xx = 0, double yy = 0) {
x = xx;
y = yy;
}
void setPoint(double xx, double yy) {
x = xx;
y = yy;
}
double getX() {
return x;
}
double getY() {
return y;
}
};
class Line : public Point {
private:
double k, b;
public:
Line(double xx = 0, double yy = 0, double kk = 0) : Point(xx, yy) {
k = kk;
b = y - k * x;
}
void setLine(double xx, double yy, double kk) {
x = xx;
y = yy;
k = kk;
b = y - k * x;
}
double getK() {
return k;
}
double getB() {
return b;
}
double getDistance(Line &l) {
double dis = fabs((l.getB() - b) / sqrt(1 + k * k));
return dis;
}
};
class Rect : public Line {
private:
double length, width;
public:
Rect(double xx = 0, double yy = 0, double kk = 0, double ll = 0, double ww = 0) : Line(xx, yy, kk) {
length = ll;
width = ww;
}
void setRect(double xx, double yy, double kk, double ll, double ww) {
x = xx;
y = yy;
k = kk;
b = y - k * x;
length = ll;
width = ww;
}
double getLength() {
return length;
}
double getWidth() {
return width;
}
double getPerimeter() {
double perimeter = 2 * (length + width);
return perimeter;
}
double getArea() {
double area = length * width;
return area;
}
};
int main() {
Rect r(1, 1, 1, 2, 3);
cout << "The length of the rectangular is " << r.getLength() << endl;
cout << "The width of the rectangular is " << r.getWidth() << endl;
cout << "The perimeter of the rectangular is " << r.getPerimeter() << endl;
cout << "The area of the rectangular is " << r.getArea() << endl;
return 0;
}
相关推荐
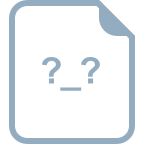
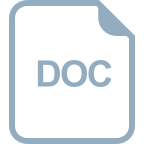
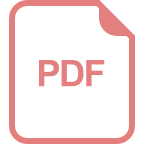















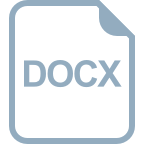