用C语言写一个算法统计二叉树的深度
时间: 2023-03-25 17:03:15 浏览: 130
您好,以下是回答您的问题:
可以使用递归的方式来统计二叉树的深度,具体实现如下:
```
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
int val;
scanf("%d", &val);
if (val == -1) {
return NULL;
}
TreeNode *root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = val;
root->left = createTree();
root->right = createTree();
return root;
}
// 统计二叉树深度
int maxDepth(TreeNode* root) {
if (root == NULL) {
return ;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
printf("请输入二叉树的节点值,-1表示空节点:\n");
TreeNode *root = createTree();
int depth = maxDepth(root);
printf("二叉树的深度为:%d\n", depth);
return ;
}
```
以上是用 C 语言写一个算法统计二叉树的深度的实现方法,希望能对您有所帮助。
阅读全文
相关推荐


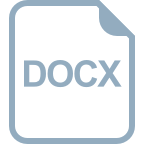
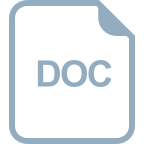
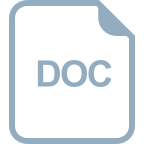
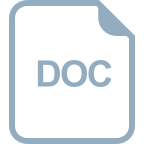
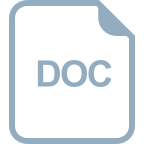
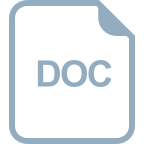







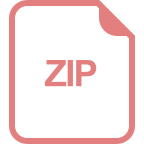