C# WPF 用MVVM框架实现鼠标操作画圆形和旋转矩形
时间: 2024-02-29 14:51:17 浏览: 24
好的,我会尽力回答你的问题。
首先,MVVM框架是一种用于构建WPF应用程序的设计模式,它将应用程序分为三个部分:模型(Model)、视图(View)和视图模型(ViewModel)。模型是应用程序的数据和业务逻辑,视图是用户界面,而视图模型是连接模型和视图的中介。
接下来,我们来实现鼠标操作画圆和旋转矩形的功能。首先,在视图模型中创建两个命令,一个用于画圆,一个用于旋转矩形。在命令的Execute方法中,我们可以通过鼠标事件获取到鼠标的位置,然后根据这个位置绘制圆或者矩形。
下面是一个简单的示例代码:
```csharp
public class MainViewModel : INotifyPropertyChanged
{
public ICommand DrawCircleCommand { get; set; }
public ICommand RotateRectangleCommand { get; set; }
private Point _lastPosition;
private ObservableCollection<Shape> _shapes = new ObservableCollection<Shape>();
public ObservableCollection<Shape> Shapes
{
get { return _shapes; }
set
{
_shapes = value;
OnPropertyChanged(nameof(Shapes));
}
}
public MainViewModel()
{
DrawCircleCommand = new RelayCommand(DrawCircle);
RotateRectangleCommand = new RelayCommand(RotateRectangle);
}
private void DrawCircle(object obj)
{
var args = obj as MouseEventArgs;
if (args != null)
{
var position = args.GetPosition(null);
var radius = 50;
var circle = new Ellipse
{
Width = radius,
Height = radius,
Fill = Brushes.Red
};
Canvas.SetLeft(circle, position.X - radius / 2);
Canvas.SetTop(circle, position.Y - radius / 2);
Shapes.Add(circle);
}
}
private void RotateRectangle(object obj)
{
var args = obj as MouseEventArgs;
if (args != null)
{
var position = args.GetPosition(null);
var width = 100;
var height = 50;
var rectangle = new Rectangle
{
Width = width,
Height = height,
Fill = Brushes.Green,
RenderTransformOrigin = new Point(0.5, 0.5)
};
Canvas.SetLeft(rectangle, position.X - width / 2);
Canvas.SetTop(rectangle, position.Y - height / 2);
var transform = new RotateTransform
{
Angle = 0
};
rectangle.RenderTransform = transform;
Shapes.Add(rectangle);
var animation = new DoubleAnimation
{
From = 0,
To = 360,
Duration = new Duration(TimeSpan.FromSeconds(5)),
RepeatBehavior = RepeatBehavior.Forever
};
transform.BeginAnimation(RotateTransform.AngleProperty, animation);
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
public class RelayCommand : ICommand
{
private Action<object> _execute;
private Func<object, bool> _canExecute;
public RelayCommand(Action<object> execute, Func<object, bool> canExecute = null)
{
_execute = execute;
_canExecute = canExecute;
}
public bool CanExecute(object parameter)
{
return _canExecute == null || _canExecute(parameter);
}
public void Execute(object parameter)
{
_execute(parameter);
}
public event EventHandler CanExecuteChanged;
public void RaiseCanExecuteChanged()
{
CanExecuteChanged?.Invoke(this, EventArgs.Empty);
}
}
```
在这个示例代码中,我们创建了一个MainViewModel类,其中包含了两个命令DrawCircleCommand和RotateRectangleCommand,分别用于绘制圆和旋转矩形。当用户在画布上单击鼠标时,会触发对应的命令,并通过鼠标事件获取到鼠标位置,然后创建相应的形状并添加到Shapes集合中。可以通过绑定Shapes集合到画布上,将绘制的形状显示在界面上。
以上是一个简单的实现,具体的实现方式可以根据自己的需求进行调整和优化。希望能对你有所帮助。
相关推荐
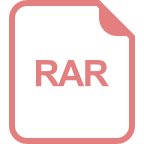
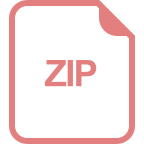
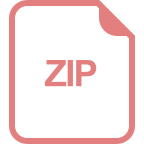














