wpf mvvmlight 鼠标改变rectangle 尺寸 控制矩形旋转
时间: 2023-12-30 19:17:34 浏览: 76
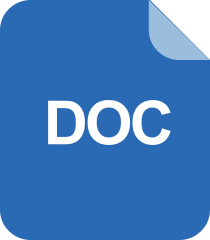
鼠标勾画矩形框改变大小
您好,针对您的问题,我可以提供以下的思路:
1. 使用WPF中的Mouse事件来监听鼠标移动和点击事件,以便控制矩形的尺寸和旋转。
2. 使用MVVM Light框架来实现数据绑定和命令绑定,以便更好地组织和管理代码。
3. 在ViewModel中定义属性和命令,将它们与View中的控件进行绑定,以便在View中显示出来并响应用户的操作。
4. 使用Transform类来实现矩形的旋转,可以通过修改矩形的旋转角度来实现。
这是一个大致的思路,具体的实现方式可以参考以下代码:
View:
```xml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:i="clr-namespace:System.Windows.Interactivity;assembly=System.Windows.Interactivity"
xmlns:cmd="clr-namespace:GalaSoft.MvvmLight.Command;assembly=GalaSoft.MvvmLight.Platform"
xmlns:local="clr-namespace:WpfApp1"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Rectangle Width="{Binding Width}" Height="{Binding Height}" Fill="Blue" Margin="100,100,0,0">
<Rectangle.RenderTransform>
<RotateTransform Angle="{Binding Angle}" CenterX="0.5" CenterY="0.5"/>
</Rectangle.RenderTransform>
<i:Interaction.Triggers>
<i:EventTrigger EventName="MouseLeftButtonDown">
<cmd:EventToCommand Command="{Binding MouseDownCommand}" PassEventArgsToCommand="True"/>
</i:EventTrigger>
<i:EventTrigger EventName="MouseMove">
<cmd:EventToCommand Command="{Binding MouseMoveCommand}" PassEventArgsToCommand="True"/>
</i:EventTrigger>
<i:EventTrigger EventName="MouseLeftButtonUp">
<cmd:EventToCommand Command="{Binding MouseUpCommand}" PassEventArgsToCommand="True"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</Rectangle>
</Grid>
</Window>
```
ViewModel:
```csharp
using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Command;
using System.Windows;
using System.Windows.Input;
namespace WpfApp1
{
public class MainViewModel : ViewModelBase
{
private double _width = 100;
private double _height = 50;
private double _angle = 0;
private Point _startPoint;
public MainViewModel()
{
MouseDownCommand = new RelayCommand<MouseButtonEventArgs>(MouseDownHandler);
MouseMoveCommand = new RelayCommand<MouseEventArgs>(MouseMoveHandler);
MouseUpCommand = new RelayCommand<MouseButtonEventArgs>(MouseUpHandler);
}
public double Width
{
get { return _width; }
set { _width = value; RaisePropertyChanged(); }
}
public double Height
{
get { return _height; }
set { _height = value; RaisePropertyChanged(); }
}
public double Angle
{
get { return _angle; }
set { _angle = value; RaisePropertyChanged(); }
}
public ICommand MouseDownCommand { get; set; }
public ICommand MouseMoveCommand { get; set; }
public ICommand MouseUpCommand { get; set; }
private void MouseDownHandler(MouseButtonEventArgs e)
{
_startPoint = e.GetPosition(null);
}
private void MouseMoveHandler(MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
Point currentPoint = e.GetPosition(null);
double deltaX = currentPoint.X - _startPoint.X;
double deltaY = currentPoint.Y - _startPoint.Y;
if (Keyboard.Modifiers == ModifierKeys.Control)
{
// 控制矩形的旋转
Angle += deltaX;
}
else
{
// 控制矩形的尺寸
Width += deltaX;
Height += deltaY;
}
_startPoint = currentPoint;
}
}
private void MouseUpHandler(MouseButtonEventArgs e)
{
_startPoint = new Point();
}
}
}
```
在这个例子中,我们使用了MVVM Light框架来实现了命令绑定和数据绑定,使用了WPF中的Mouse事件来监听鼠标移动和点击事件,使用了Transform类来实现了矩形的旋转。通过以上的代码,可以实现鼠标改变矩形尺寸和旋转的功能。
阅读全文
相关推荐







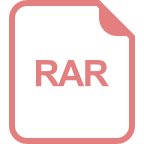
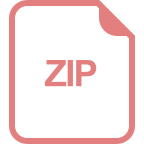
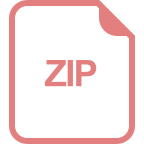





