pca算法的完整python代码实现
时间: 2023-07-25 08:43:36 浏览: 109
以下是PCA算法的Python代码实现:
```python
import numpy as np
def pca(X, n_components):
"""
实现PCA算法
:param X: 输入矩阵,每一行表示一个样本
:param n_components: 保留的主成分个数
:return: 降维后的矩阵
"""
# 将样本矩阵按列中心化
mean = np.mean(X, axis=0)
X_centered = X - mean
# 计算样本矩阵的协方差矩阵
cov_matrix = np.cov(X_centered.T)
# 计算协方差矩阵的特征值和特征向量
eigenvalues, eigenvectors = np.linalg.eig(cov_matrix)
# 将特征向量按特征值从大到小排序
sorted_indices = np.argsort(eigenvalues)[::-1]
sorted_eigenvectors = eigenvectors[:, sorted_indices]
# 选取前n_components个特征向量组成投影矩阵
projection_matrix = sorted_eigenvectors[:, :n_components]
# 将样本矩阵投影到新的低维空间
X_reduced = np.dot(X_centered, projection_matrix)
return X_reduced
```
使用示例:
```python
import numpy as np
# 生成100个二维随机样本
X = np.random.rand(100, 2)
# 调用PCA算法进行降维
X_reduced = pca(X, n_components=1)
# 输出降维后的矩阵形状
print(X_reduced.shape) # (100, 1)
```
阅读全文
相关推荐
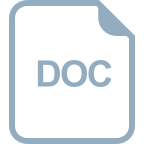
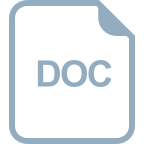
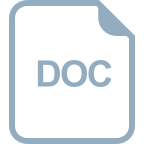
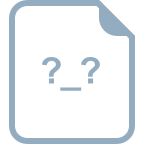
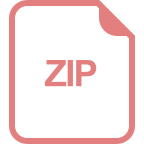
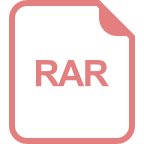
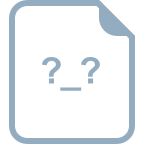
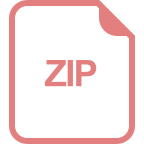
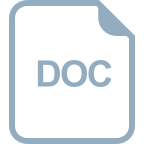





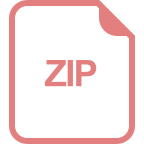



