采用链表数据结构实现一“图书管理系统〞,为用户提供管理和查询服务。 图书信息包括8个数据项:书名,ISBN、作者、出版社、出版时间,入库时间, 库存量(图书馆购买此书的数量),借阅数(被借阅的数量)等信息。完成以下 操作: (1)录入:图书信息(至少10条记录): (2)删除:删除某一本图书的信息; (3)修改:修改某一本图书的信息: (4)查询:根据书名或图书ISBV等查询图书信息(查询方法不限)。 (5)借书:借出一本书,该书的借阅数增加一本。 (6)还书:还回一本书,该书的借阅数减少一本。 (7)统计:统计所有借出的图书数目,即所有图书借阅数之和。 (8)输出:输出所查询图书的信息,要求格式清晰,方便查看。
时间: 2023-11-28 12:49:24 浏览: 23
这是一道比较典型的图书管理系统的编程题目,可以用链表数据结构来实现。下面是一份C++示例代码,供您参考:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义图书信息结构体
struct BookInfo {
string bookName; // 书名
string ISBN; // ISBN
string author; // 作者
string publisher; // 出版社
string publishTime; // 出版时间
string storeTime; // 入库时间
int storage; // 库存量
int borrow; // 借阅数
BookInfo* next; // 下一本书的指针
};
// 定义图书管理系统类
class BookManager {
public:
BookManager() {
head = NULL;
bookCount = 0;
}
~BookManager() {
BookInfo* current = head;
while (current != NULL) {
BookInfo* temp = current->next;
delete current;
current = temp;
}
}
// 添加图书信息
void addBookInfo() {
BookInfo* book = new BookInfo;
cout << "请输入书名:";
cin >> book->bookName;
cout << "请输入ISBN:";
cin >> book->ISBN;
cout << "请输入作者:";
cin >> book->author;
cout << "请输入出版社:";
cin >> book->publisher;
cout << "请输入出版时间:";
cin >> book->publishTime;
cout << "请输入入库时间:";
cin >> book->storeTime;
cout << "请输入库存量:";
cin >> book->storage;
cout << "请输入借阅数:";
cin >> book->borrow;
book->next = NULL;
if (head == NULL) {
head = book;
}
else {
BookInfo* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = book;
}
bookCount++;
cout << "添加成功!" << endl;
}
// 删除图书信息
void deleteBookInfo() {
string bookName;
cout << "请输入要删除的书名:";
cin >> bookName;
BookInfo* current = head;
BookInfo* prev = NULL;
while (current != NULL) {
if (current->bookName == bookName) {
if (prev == NULL) {
head = current->next;
}
else {
prev->next = current->next;
}
delete current;
bookCount--;
cout << "删除成功!" << endl;
return;
}
prev = current;
current = current->next;
}
cout << "未找到该书籍!" << endl;
}
// 修改图书信息
void modifyBookInfo() {
string bookName;
cout << "请输入要修改的书名:";
cin >> bookName;
BookInfo* current = head;
while (current != NULL) {
if (current->bookName == bookName) {
cout << "请输入新的ISBN:";
cin >> current->ISBN;
cout << "请输入新的作者:";
cin >> current->author;
cout << "请输入新的出版社:";
cin >> current->publisher;
cout << "请输入新的出版时间:";
cin >> current->publishTime;
cout << "请输入新的入库时间:";
cin >> current->storeTime;
cout << "请输入新的库存量:";
cin >> current->storage;
cout << "请输入新的借阅数:";
cin >> current->borrow;
cout << "修改成功!" << endl;
return;
}
current = current->next;
}
cout << "未找到该书籍!" << endl;
}
// 查询图书信息
void queryBookInfo() {
string keyword;
cout << "请输入查询关键字:";
cin >> keyword;
BookInfo* current = head;
while (current != NULL) {
if (current->bookName == keyword || current->ISBN == keyword) {
cout << "书名:" << current->bookName << endl;
cout << "ISBN:" << current->ISBN << endl;
cout << "作者:" << current->author << endl;
cout << "出版社:" << current->publisher << endl;
cout << "出版时间:" << current->publishTime << endl;
cout << "入库时间:" << current->storeTime << endl;
cout << "库存量:" << current->storage << endl;
cout << "借阅数:" << current->borrow << endl;
return;
}
current = current->next;
}
cout << "未找到该书籍!" << endl;
}
// 借出图书
void borrowBook() {
string bookName;
cout << "请输入要借阅的书名:";
cin >> bookName;
BookInfo* current = head;
while (current != NULL) {
if (current->bookName == bookName) {
if (current->storage > 0) {
current->storage--;
current->borrow++;
cout << "借阅成功!" << endl;
return;
}
else {
cout << "库存不足,无法借阅!" << endl;
return;
}
}
current = current->next;
}
cout << "未找到该书籍!" << endl;
}
// 归还图书
void returnBook() {
string bookName;
cout << "请输入要归还的书名:";
cin >> bookName;
BookInfo* current = head;
while (current != NULL) {
if (current->bookName == bookName) {
if (current->borrow > 0) {
current->borrow--;
current->storage++;
cout << "归还成功!" << endl;
return;
}
else {
cout << "该书未被借出,无法归还!" << endl;
return;
}
}
current = current->next;
}
cout << "未找到该书籍!" << endl;
}
// 统计借出的图书数目
void countBorrow() {
int totalBorrow = 0;
BookInfo* current = head;
while (current != NULL) {
totalBorrow += current->borrow;
current = current->next;
}
cout << "借出的图书数目为:" << totalBorrow << endl;
}
private:
BookInfo* head; // 头节点指针
int bookCount; // 图书数量
};
int main() {
BookManager manager;
int choice = 0;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加图书信息" << endl;
cout << "2. 删除图书信息" << endl;
cout << "3. 修改图书信息" << endl;
cout << "4. 查询图书信息" << endl;
cout << "5. 借出图书" << endl;
cout << "6. 归还图书" << endl;
cout << "7. 统计借出的图书数目" << endl;
cout << "0. 退出" << endl;
cin >> choice;
switch (choice) {
case 1:
manager.addBookInfo();
break;
case 2:
manager.deleteBookInfo();
break;
case 3:
manager.modifyBookInfo();
break;
case 4:
manager.queryBookInfo();
break;
case 5:
manager.borrowBook();
break;
case 6:
manager.returnBook();
break;
case 7:
manager.countBorrow();
break;
case 0:
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
在这份代码中,我们定义了一个 `BookManager` 类来实现图书管理系统。在类中,我们使用了链表数据结构来存储图书信息,每本书的信息都被封装在一个 `BookInfo` 结构体中,同时包含了一个指向下一本书的指针。在类中,我们实现了添加、删除、修改、查询、借出、归还、统计等操作,用户可以通过终端输入来进行操作。
相关推荐
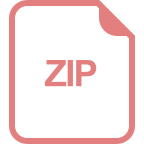
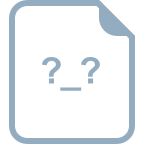
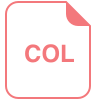
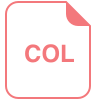
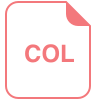
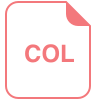
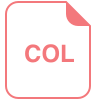







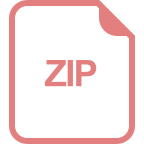
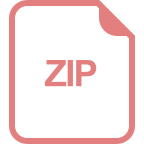
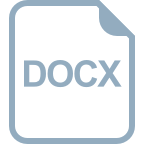
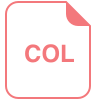