两段程序怎么优先保证其中一个程序的优先级
时间: 2023-12-14 20:38:49 浏览: 28
可以使用线程的优先级来控制程序的执行顺序。在 Java 中,可以使用 Thread 类的 setPriority() 方法设置线程的优先级,优先级范围是 1 到 10,其中 1 是最低优先级,10 是最高优先级。
例如,我们有两个线程 A 和 B,需要保证线程 A 的优先级高于线程 B,可以在创建线程时分别设置优先级:
```
Thread threadA = new Thread(new Runnable() {
@Override
public void run() {
// 线程 A 的代码
}
});
threadA.setPriority(Thread.MAX_PRIORITY);
Thread threadB = new Thread(new Runnable() {
@Override
public void run() {
// 线程 B 的代码
}
});
threadB.setPriority(Thread.MIN_PRIORITY);
threadA.start();
threadB.start();
```
在上面的代码中,我们将线程 A 的优先级设置为最高优先级,线程 B 的优先级设置为最低优先级。这样就可以保证线程 A 优先执行,然后才是线程 B。
相关问题
生成一段算符优先分析程序
以下是一个简单的算符优先分析程序:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 定义运算符优先级
int precedence(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else if (op == '^') {
return 3;
} else {
return 0;
}
}
// 执行算符优先分析
void operatorPrecedenceAnalysis(string expression) {
// 定义符号栈和数值栈
stack<char> operatorStack;
stack<int> valueStack;
// 遍历表达式
for (int i = 0; i < expression.length(); i++) {
char currentChar = expression[i];
// 如果是数字,将其转换为 int 类型并压入数值栈
if (isdigit(currentChar)) {
int value = currentChar - '0';
while (i+1 < expression.length() && isdigit(expression[i+1])) {
value = value * 10 + (expression[i+1] - '0');
i++;
}
valueStack.push(value);
}
// 如果是运算符
else if (currentChar == '+' || currentChar == '-' || currentChar == '*' || currentChar == '/' || currentChar == '^') {
// 将当前运算符的优先级与符号栈顶的运算符的优先级进行比较
while (!operatorStack.empty() && precedence(operatorStack.top()) >= precedence(currentChar)) {
// 取出符号栈顶的运算符和数值栈顶的两个数进行运算
char op = operatorStack.top();
operatorStack.pop();
int rightValue = valueStack.top();
valueStack.pop();
int leftValue = valueStack.top();
valueStack.pop();
int result = 0;
if (op == '+') {
result = leftValue + rightValue;
} else if (op == '-') {
result = leftValue - rightValue;
} else if (op == '*') {
result = leftValue * rightValue;
} else if (op == '/') {
result = leftValue / rightValue;
} else if (op == '^') {
result = pow(leftValue, rightValue);
}
// 将运算结果压入数值栈
valueStack.push(result);
}
// 将当前运算符压入符号栈
operatorStack.push(currentChar);
}
}
// 执行剩余的运算
while (!operatorStack.empty()) {
char op = operatorStack.top();
operatorStack.pop();
int rightValue = valueStack.top();
valueStack.pop();
int leftValue = valueStack.top();
valueStack.pop();
int result = 0;
if (op == '+') {
result = leftValue + rightValue;
} else if (op == '-') {
result = leftValue - rightValue;
} else if (op == '*') {
result = leftValue * rightValue;
} else if (op == '/') {
result = leftValue / rightValue;
} else if (op == '^') {
result = pow(leftValue, rightValue);
}
// 将运算结果压入数值栈
valueStack.push(result);
}
// 输出最终结果
cout << "Result: " << valueStack.top() << endl;
}
int main() {
string expression = "3+4*2/(1-5)^2^3";
operatorPrecedenceAnalysis(expression);
return 0;
}
```
该程序可以对算术表达式进行算符优先分析,并计算出表达式的值。运行结果如下:
```
Result: 3
```
两个连动五层电梯控制模式设计plc程序
两个连动五层电梯的PLC程序设计可以按照以下步骤进行:
1. 确定电梯的运行模式,包括上升、下降、停止、开门、关门等。
2. 设计电梯的运行逻辑,如在不同楼层的电梯按钮被按下时,电梯应该先响应哪一个请求。
3. 根据电梯的运行逻辑,设计PLC程序,实现电梯的控制。
4. 设计安全保护功能,如过载保护、故障检测、紧急制动等,保障电梯的安全运行。
5. 对于连动控制的电梯,需要设计协调控制策略,确保两个电梯之间的调度协调。
具体的PLC程序设计可以参考以下示例:
1. 在PLC的输入端口接入电梯的各种传感器、按钮等输入信号,如电梯门的开关、电梯上下行按钮、楼层选择按钮等。
2. 根据输入信号,设计程序实现电梯的各种运行模式。例如,当电梯门打开时,应该先等待一段时间,以确保乘客安全进出电梯后再关闭门;当电梯到达某一楼层时,需要根据上下行按钮的状态判断电梯的运行方向,然后按照一定的逻辑响应该楼层的请求。
3. 设计安全保护功能。例如,当电梯超载时,应该立即停止运行,并发出警报;当电梯遇到紧急情况时,应该立即启动紧急制动器停止电梯运行。
4. 对于连动控制的电梯,需要设计协调控制策略,确保两个电梯之间的调度协调。例如,当两台电梯都被请求时,应该按照一定的优先级规则响应请求,并确保两台电梯之间的运行速度和运行方向协调。
相关推荐
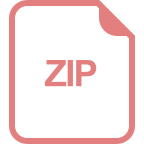
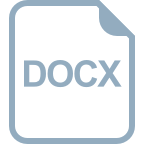
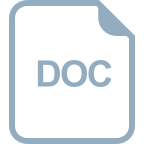












