用python将excel导入neo4j
时间: 2023-05-31 20:18:28 浏览: 908
### 回答1:
可以使用Python的pandas库来读取Excel文件,并使用py2neo库将数据导入Neo4j数据库。
以下是大致的步骤:
1. 安装pandas和py2neo库
可以使用pip命令来安装:
```
pip install pandas py2neo
```
2. 读取Excel文件
使用pandas库的read_excel函数来读取Excel文件,例如:
```
import pandas as pd
df = pd.read_excel('data.xlsx')
```
其中,'data.xlsx'是Excel文件的路径。
3. 连接Neo4j数据库
使用py2neo库来连接Neo4j数据库,例如:
```
from py2neo import Graph, Node, Relationship
graph = Graph("bolt://localhost:7687", auth=("neo4j", "password"))
```
其中,"bolt://localhost:7687"是Neo4j数据库的地址和端口,"neo4j"和"password"是数据库的用户名和密码。
4. 将数据导入Neo4j数据库
使用py2neo库来创建节点和关系,并将它们添加到Neo4j数据库中,例如:
```
for index, row in df.iterrows():
node1 = Node("Label1", name=row['Column1'])
node2 = Node("Label2", name=row['Column2'])
rel = Relationship(node1, "REL_TYPE", node2)
graph.create(node1)
graph.create(node2)
graph.create(rel)
```
其中,"Label1"和"Label2"是节点的标签,"Column1"和"Column2"是Excel文件中的列名,"REL_TYPE"是关系的类型。
完成以上步骤后,就可以将Excel文件中的数据导入到Neo4j数据库中了。
### 回答2:
Python是一种广泛用于数据分析和处理的编程语言,而Neo4j是一种用于图形数据库的开源产品。将Excel数据导入Neo4j可以让用户使用Neo4j的高级图形查询功能,来挖掘数据之间的关系以及进行高效的数据分析。本文将演示如何使用Python从Excel文件中导入数据到Neo4j数据库。
首先,需要安装一些必需的库。我们可以使用pip package installer在命令行中输入以下代码来安装所需的库,如下:
pip install pandas #数据分析库
pip install neo4j #Neo4j数据库连接库
pip install xlrd #Excel读取库
在完成库的安装后,我们需要建立一个Neo4j数据库的连接。我们将创建一个函数,用于指定数据库的URL、用户名和密码并建立连接。
```python
from neo4j import GraphDatabase
def create_connection():
url = "bolt://localhost:7687" #Neo4j数据库的URL
username = "neo4j" #Neo4j数据库的用户名
password = "yourpassword" #Neo4j数据库的密码
driver = GraphDatabase.driver(url, auth=(username, password))
return driver.session()
```
接下来,我们需要读取Excel文件中的数据。我们可以使用pandas库中的read_excel()函数来读取Excel文件,如下:
```python
import pandas as pd
def read_excel(file_path):
df = pd.read_excel(file_path)
return df
```
读取Excel文件后,我们可以对数据进行处理以适应Neo4j数据库的结构。在Neo4j中,节点是由标签和属性组成的,而关系是由名称和属性组成的。我们需要将数据转换为节点和关系,并将其插入到Neo4j数据库中。过程如下:
```python
def insert_to_db(tx, name, attributes):
# 格式化属性为Cypher查询语句格式
props = ','.join(f"{key}: '{value}'" for key, value in attributes.items())
query = f"CREATE (: {name} {{{props}}})"
tx.run(query)
def insert_relationship(tx, source_node_id, target_node_id, name, attributes):
#格式化属性为Cypher查询语句格式
props = ','.join(f"{key}:'{value}'" for key, value in attributes.items())
query = f"MATCH (a), (b) WHERE a.id = '{source_node_id}' AND b.id = '{target_node_id}' CREATE(a) - [:{name} {{{props}}}]->(b)"
tx.run(query)
def save_to_neo4j(df):
with create_connection() as session:
nodes = set()
relationships = set()
for row in df.itertuples():
source_id = getattr(row, "source_id")
target_id = getattr(row, "target_id")
relation_type = getattr(row, "relation_type")
source_node_type = getattr(row, "source_node_type")
target_node_type = getattr(row, "target_node_type")
source_attributes = dict(row._asdict())
del source_attributes["source_id"]
del source_attributes["target_id"]
del source_attributes["relation_type"]
del source_attributes["source_node_type"]
del source_attributes["target_node_type"]
target_attributes = source_attributes.copy()
del target_attributes["name"]
source_node = (source_node_type, source_id, source_attributes)
target_node = (target_node_type, target_id, target_attributes)
relationship = (relation_type, source_id, target_id, source_attributes)
nodes.add(source_node)
nodes.add(target_node)
relationships.add(relationship)
for node in nodes:
insert_to_db(session.write_transaction, node[0], node[2])
for relationship in relationships:
insert_relationship(session.write_transaction, relationship[1], relationship[2], relationship[0], relationship[3])
```
通过运行以上代码,我们已经成功将Excel中的数据导入到Neo4j数据库中了。现在,用户可以使用Neo4j的高级图形查询语言(Cypher)来分析从Excel中导入的数据之间的关系。该方法可以帮助用户快速有效地进行数据分析和数据挖掘。
综上所述,通过Python将Excel数据导入Neo4j数据库是一种方便、高效的数据处理方法。以上是将Excel数据导入Neo4j的基本步骤,用户可以根据自身需求自定义参数、关系、标签等细节。
### 回答3:
Neo4j是一种基于图形数据库的工具,可用于存储、分析和查询高度联通的数据。在实际应用中,可以用Python编写代码将Excel文件导入Neo4j数据库中。本文将讨论如何使用Python将Excel文件导入Neo4j数据库。此外,Neo4j的Python驱动程序可以让我们轻松地连接到本地或远程Neo4j服务器。
在Neo4j中,数据结构是一个节点与节点之间的关系的网络。因此,在导入Excel时,我们需要先定义如何将Excel中的数据映射到Neo4j中的节点和关系。下面是Python代码实现的过程,步骤如下:
1. 安装Py2neo库:Py2neo是Python的Neo4j驱动程序。我们可以使用pip从命令行安装它,安装命令如下:
pip install py2neo
2. 创建节点:在我们导入Excel之前,我们需要定义如何将数据映射到节点。例如,一个人可以被表示为一个节点,其中节点的属性包括姓名、年龄、性别等。以下是如何创建一个人节点的Python代码:
from py2neo import Graph, Node
graph = Graph("bolt://localhost:7687", auth=("username", "password"))
person = Node("Person", name="John", age=30, gender="Male")
tx = graph.begin()
tx.create(person)
tx.commit()
3. 创建关系:在Neo4j中,两个节点之间的关系也是一个节点,因此我们需要定义如何将Excel数据映射到关系节点。例如,关系可以是两个人之间的朋友关系,我们可以将其表示为一个关系节点,并将其属性设置为开始节点、结束节点和关系类型。以下是如何创建一个朋友节点的Python代码:
from py2neo import Graph, Node
graph = Graph("bolt://localhost:7687", auth=("username", "password"))
person1 = Node("Person", name="John", age=30, gender="Male")
person2 = Node("Person", name="Jane", age=25, gender="Female")
friendship = Node("Friendship", type="Friend", start_node=person1, end_node=person2)
tx = graph.begin()
tx.create(person1)
tx.create(person2)
tx.create(friendship)
tx.commit()
4. 从Excel中读取数据:我们可以使用Python的openpyxl库来读取Excel文件。例如,以下Python代码将打开一个名为“data.xlsx”的Excel文件并读取第一个工作表中的所有行和列的数据:
import openpyxl
workbook = openpyxl.load_workbook("data.xlsx")
sheet = workbook.active
for row in sheet.iter_rows():
for cell in row:
print(cell.value)
5. 将数据插入到Neo4j中:当我们从Excel读取数据并将其映射到节点和关系时,我们可以使用Py2neo库将其插入到Neo4j中。例如,以下Python代码将从Excel文件“data.xlsx”中的第一列和第二列读取数据,并将其映射到两个节点和一个关系节点:
from py2neo import Graph, Node
graph = Graph("bolt://localhost:7687", auth=("username", "password"))
workbook = openpyxl.load_workbook("data.xlsx")
sheet = workbook.active
for row in sheet.iter_rows():
person1 = Node("Person", name=row[0].value)
person2 = Node("Person", name=row[1].value)
friendship = Node("Friendship", type="Friend", start_node=person1, end_node=person2)
tx = graph.begin()
tx.create(person1)
tx.create(person2)
tx.create(friendship)
tx.commit()
在实现导入Excel并将数据插入到Neo4j中的过程中,需要注意以下细节:
1. Excel中的数据必须经过适当的清洗和格式化,以便正确地映射到节点和关系。
2. 如果从外部源导入数据,则必须确保有添加节点和关系的必要权限。
3. Neo4j服务器必须处于运行状态,才能正确地将Excel数据插入到数据库中。
综上,Python可以用来将Excel文件导入Neo4j数据库中,我们需要定义如何将Excel中的数据映射到Neo4j中的节点和关系,使用openpyxl库来读取Excel数据,使用Py2neo库将它们插入到Neo4j中。这种数据导入方法可以帮助我们更好地理解和分析高度联通的数据。
阅读全文
相关推荐
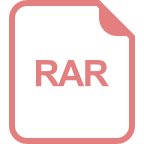













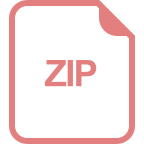
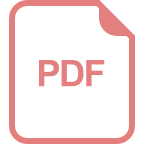