写一段围棋判断胜负的C语言代码
时间: 2024-05-11 11:19:54 浏览: 141
以下是一个简单的围棋判断胜负的C语言代码示例:
```c
#include <stdio.h>
#define BOARD_SIZE 19
enum COLOR { EMPTY, BLACK, WHITE };
int board[BOARD_SIZE][BOARD_SIZE]; // 存储棋盘状态
int current_player = BLACK; // 当前玩家
// 判断是否越界
int is_valid_position(int x, int y) {
return x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE;
}
// 判断坐标位置是否为空
int is_empty_position(int x, int y) {
return board[x][y] == EMPTY;
}
// 判断当前位置是否为当前玩家的棋子
int is_current_player_piece(int x, int y) {
return board[x][y] == current_player;
}
// 判断当前位置是否为对手的棋子
int is_opponent_piece(int x, int y) {
return board[x][y] == (current_player == BLACK ? WHITE : BLACK);
}
// 判断当前位置是否为活棋眼
int is_live_eye(int x, int y) {
// 活棋眼的定义:周围有 4 个空位,并且当前玩家的棋子全部在周围
if (board[x][y] != EMPTY) {
return 0;
}
int num_empty = 0, num_current_player = 0;
if (is_valid_position(x - 1, y) && is_empty_position(x - 1, y)) {
num_empty++;
} else if (is_valid_position(x - 1, y) && is_current_player_piece(x - 1, y)) {
num_current_player++;
}
if (is_valid_position(x + 1, y) && is_empty_position(x + 1, y)) {
num_empty++;
} else if (is_valid_position(x + 1, y) && is_current_player_piece(x + 1, y)) {
num_current_player++;
}
if (is_valid_position(x, y - 1) && is_empty_position(x, y - 1)) {
num_empty++;
} else if (is_valid_position(x, y - 1) && is_current_player_piece(x, y - 1)) {
num_current_player++;
}
if (is_valid_position(x, y + 1) && is_empty_position(x, y + 1)) {
num_empty++;
} else if (is_valid_position(x, y + 1) && is_current_player_piece(x, y + 1)) {
num_current_player++;
}
return num_empty == 4 && num_current_player == 4;
}
// 判断当前位置是否为死棋眼
int is_dead_eye(int x, int y) {
// 死棋眼的定义:周围有 4 个空位,并且当前玩家的棋子不全在周围
if (board[x][y] != EMPTY) {
return 0;
}
int num_empty = 0, num_current_player = 0;
if (is_valid_position(x - 1, y) && is_empty_position(x - 1, y)) {
num_empty++;
} else if (is_valid_position(x - 1, y) && is_current_player_piece(x - 1, y)) {
num_current_player++;
}
if (is_valid_position(x + 1, y) && is_empty_position(x + 1, y)) {
num_empty++;
} else if (is_valid_position(x + 1, y) && is_current_player_piece(x + 1, y)) {
num_current_player++;
}
if (is_valid_position(x, y - 1) && is_empty_position(x, y - 1)) {
num_empty++;
} else if (is_valid_position(x, y - 1) && is_current_player_piece(x, y - 1)) {
num_current_player++;
}
if (is_valid_position(x, y + 1) && is_empty_position(x, y + 1)) {
num_empty++;
} else if (is_valid_position(x, y + 1) && is_current_player_piece(x, y + 1)) {
num_current_player++;
}
return num_empty == 4 && num_current_player != 4;
}
// 判断当前位置是否为目
int is_eye(int x, int y) {
return is_live_eye(x, y) || is_dead_eye(x, y);
}
// 判断当前玩家是否胜利
int is_current_player_win() {
// 如果对手没有有效着法,当前玩家胜利
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (is_current_player_piece(i, j)) {
if (is_eye(i, j)) {
continue;
}
if (is_valid_position(i - 1, j) && is_empty_position(i - 1, j)) {
return 0;
}
if (is_valid_position(i + 1, j) && is_empty_position(i + 1, j)) {
return 0;
}
if (is_valid_position(i, j - 1) && is_empty_position(i, j - 1)) {
return 0;
}
if (is_valid_position(i, j + 1) && is_empty_position(i, j + 1)) {
return 0;
}
}
}
}
return 1;
}
int main() {
// 初始化棋盘状态
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
// 依次落子并判断胜负
board[3][3] = BLACK;
board[3][4] = WHITE;
board[4][3] = WHITE;
board[4][4] = BLACK;
current_player = BLACK;
if (is_current_player_win()) {
printf("Black wins!\n");
} else {
printf("White wins!\n");
}
return 0;
}
```
该示例代码实现了一个简单的围棋判断胜负的功能,具体实现方式如下:
1. 定义了棋盘状态的数据结构 `board`,使用枚举类型 `COLOR` 表示棋子的颜色。
2. 实现了一些辅助函数,包括判断坐标是否越界、判断当前位置是否为空、判断当前位置是否为当前玩家的棋子、判断当前位置是否为对手的棋子、判断当前位置是否为活棋眼、判断当前位置是否为死棋眼、判断当前位置是否为目等。
3. 实现了判断当前玩家是否胜利的函数 `is_current_player_win()`,该函数遍历棋盘上所有当前玩家的棋子,判断每个棋子是否在活棋眼中,如果不在,则判断周围是否有空位,如果有,则对手有有效着法,当前玩家没有胜利;如果没有,则对手没有有效着法,当前玩家胜利。
4. 在 `main()` 函数中,依次落子并调用 `is_current_player_win()` 函数判断胜负,输出胜利者的颜色。
需要注意的是,该示例代码还比较简单,只考虑了最基本的情况,实际上围棋的胜负判断还有很多复杂的情况需要考虑,例如提子、劫争等,这些情况需要在代码中进行特殊处理。
阅读全文
相关推荐
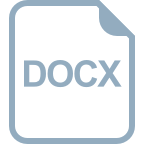
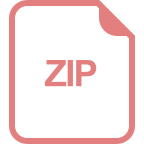












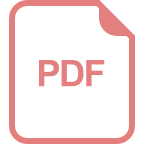
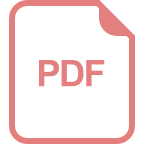