使用Directional Lifting Wavelet Transform处理图像的python代码及其逆变换
时间: 2023-12-10 20:37:46 浏览: 126
Directional Lifting Wavelet Transform (DLWT) 是一种基于 lifting scheme 的小波变换,它的特点是在小波系数的计算过程中,引入了方向信息。以下是一个使用 DLWT 处理图像的 Python 代码及其逆变换。
```python
import numpy as np
from scipy import signal
def dlwt2(img, level):
"""
将二维图像进行 Directional Lifting Wavelet Transform
:param img: 二维图像,numpy 数组,dtype 为 float
:param level: 分解层数
:return: 分解后的系数,一个包含 level+1 个 numpy 数组的列表,每个数组的 dtype 都为 float
"""
# 定义水平和垂直方向的滤波器
h_filter = np.array([1, 2, 1]) / np.sqrt(2)
v_filter = np.array([-1, 0, 1]) / np.sqrt(2)
# 定义两个对角方向的滤波器
d_filter1 = np.array([1, -2, 1]) / np.sqrt(6)
d_filter2 = np.array([-1, 2, 1]) / np.sqrt(6)
# 将图像转换为 float 类型
img = img.astype(np.float64)
# 定义分解后的系数列表
coeffs = [img]
# 进行 level 层分解
for i in range(level):
# 对每一行进行滤波
for j in range(img.shape[0]):
img[j] = signal.convolve(img[j], h_filter, mode='same', method='direct')
# 对每一列进行滤波
for k in range(img.shape[1]):
img[:, k] = signal.convolve(img[:, k], v_filter, mode='same', method='direct')
# 对两个对角方向进行滤波
for j in range(img.shape[0] - 2):
for k in range(img.shape[1] - 2):
img[j + 1, k + 1] += d_filter1.dot([img[j, k + 1], img[j + 1, k], img[j + 2, k + 1]])
img[j + 1, k + 1] += d_filter2.dot([img[j, k], img[j + 1, k + 1], img[j + 2, k + 2]])
# 将处理后的图像保存到系数列表中
coeffs.append(img.copy())
return coeffs
def idlwt2(coeffs):
"""
将 DLWT 的分解系数进行反变换,恢复原始图像
:param coeffs: 分解系数,一个包含 level+1 个 numpy 数组的列表,每个数组的 dtype 都为 float
:return: 恢复的原始图像,numpy 数组,dtype 为 float
"""
# 定义水平和垂直方向的滤波器
h_filter = np.array([1, 2, 1]) / np.sqrt(2)
v_filter = np.array([-1, 0, 1]) / np.sqrt(2)
# 定义两个对角方向的滤波器
d_filter1 = np.array([1, -2, 1]) / np.sqrt(6)
d_filter2 = np.array([-1, 2, 1]) / np.sqrt(6)
# 取出最后一层的系数,作为初始图像
img = coeffs[-1].copy()
# 从最后一层开始逐层反向处理
for i in range(len(coeffs) - 2, -1, -1):
# 对两个对角方向进行逆滤波
for j in range(coeffs[i + 1].shape[0] - 2):
for k in range(coeffs[i + 1].shape[1] - 2):
img[j, k + 1] -= d_filter1.dot([img[j + 1, k + 1], coeffs[i + 1][j, k + 1], img[j + 2, k + 1]])
img[j + 2, k + 1] -= d_filter2.dot([img[j + 1, k], coeffs[i + 1][j + 2, k + 1], img[j, k + 1]])
# 对每一列进行逆滤波
for k in range(coeffs[i + 1].shape[1]):
img[:, k] -= signal.convolve(coeffs[i + 1][:, k], v_filter[::-1], mode='same', method='direct')
# 对每一行进行逆滤波
for j in range(coeffs[i + 1].shape[0]):
img[j] -= signal.convolve(coeffs[i + 1][j], h_filter[::-1], mode='same', method='direct')
# 将图像转换为 float 类型
img = img.astype(np.float64)
return img
```
使用示例:
```python
import cv2
import matplotlib.pyplot as plt
# 读取图像
img = cv2.imread('lena.jpg', cv2.IMREAD_GRAYSCALE)
# 进行 DLWT 分解
coeffs = dlwt2(img, 3)
# 对分解后的系数进行处理,例如可以将高频系数置零,然后再进行逆变换
coeffs[1:] = [np.zeros_like(c) for c in coeffs[1:]]
reconstructed_img = idlwt2(coeffs)
# 显示原始图像和恢复的图像
plt.subplot(121)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.subplot(122)
plt.imshow(reconstructed_img, cmap='gray')
plt.title('Reconstructed Image')
plt.show()
```
阅读全文
相关推荐
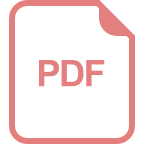
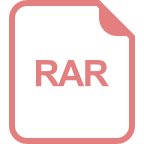



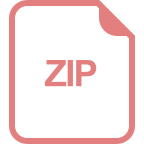
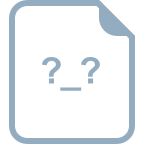
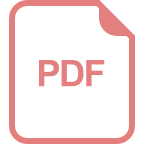
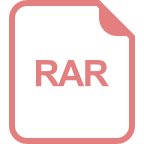
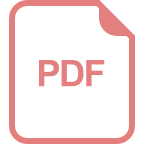
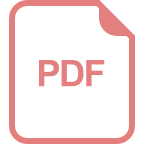
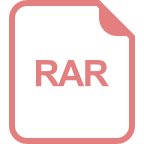
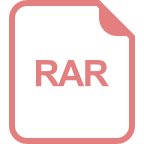
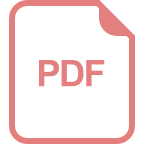
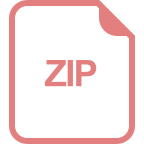
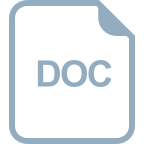
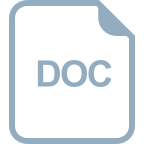