用iostream编写c++程序从键盘上输入若干学生的成绩,统计并输出最高成绩和最低成绩,当输入负数时结束输入
时间: 2023-05-17 22:06:23 浏览: 104
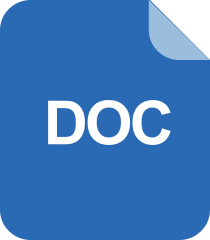
C语言输入学生成绩,计算并输出这些学生的最低分、最高分、平均分。
以下是C知道的回答:
可以使用以下代码实现:
```c
#include <stdio.h>
int main() {
int score, max_score = -1, min_score = 101;
printf("请输入学生的成绩:\n");
while (1) {
scanf("%d", &score);
if (score < 0) {
break;
}
if (score > max_score) {
max_score = score;
}
if (score < min_score) {
min_score = score;
}
}
printf("最高成绩为:%d\n", max_score);
printf("最低成绩为:%d\n", min_score);
return 0;
}
```
这个程序使用了一个 while 循环来不断读入学生的成绩,当输入负数时结束输入。在循环中,使用 if 语句来统计最高成绩和最低成绩。最后,使用 printf 函数输出结果。
希望这个回答能够帮助到你!
阅读全文
相关推荐
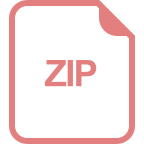











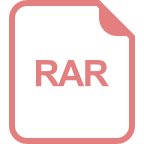
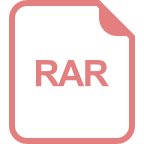
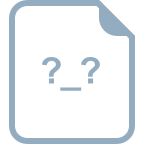
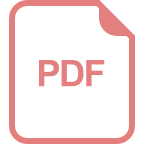
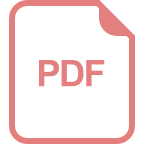