图书借阅程序示例,其中包含添加书籍、借阅书籍和归还书籍,借阅人信息及查询的功能
时间: 2023-11-27 14:54:35 浏览: 141
这是一个简单的图书借阅程序示例,包含添加书籍、借阅书籍和归还书籍,借阅人信息及查询的功能。代码使用 Python 语言编写。
```python
class Book:
def __init__(self, title, author, ISBN, num_copies):
self.title = title
self.author = author
self.ISBN = ISBN
self.num_copies = num_copies
self.num_borrowed = 0
def borrow(self):
if self.num_borrowed < self.num_copies:
self.num_borrowed += 1
return True
else:
return False
def return_book(self):
if self.num_borrowed > 0:
self.num_borrowed -= 1
return True
else:
return False
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def borrow_book(self, title):
for book in self.books:
if book.title == title:
if book.borrow():
return True
else:
return False
return False
def return_book(self, title):
for book in self.books:
if book.title == title:
if book.return_book():
return True
else:
return False
return False
def search_book(self, title):
for book in self.books:
if book.title == title:
return book
return None
class Borrower:
def __init__(self, name, ID):
self.name = name
self.ID = ID
self.borrowed_books = []
def borrow_book(self, book):
if book.borrow():
self.borrowed_books.append(book)
return True
else:
return False
def return_book(self, book):
if book.return_book():
self.borrowed_books.remove(book)
return True
else:
return False
def search_borrowed_books(self, title):
for book in self.borrowed_books:
if book.title == title:
return book
return None
```
使用示例:
```python
# 创建书籍
book1 = Book("The Great Gatsby", "F. Scott Fitzgerald", "9781847496140", 5)
book2 = Book("To Kill a Mockingbird", "Harper Lee", "9780446310789", 3)
book3 = Book("1984", "George Orwell", "9780141036144", 2)
# 创建图书馆
library = Library()
# 添加书籍
library.add_book(book1)
library.add_book(book2)
library.add_book(book3)
# 借阅书籍
library.borrow_book("1984")
# 归还书籍
library.return_book("1984")
# 查询书籍
book = library.search_book("The Great Gatsby")
# 创建借阅者
borrower = Borrower("John Smith", "123456")
# 借阅书籍
borrower.borrow_book(book1)
# 查询借阅的书籍
borrowed_book = borrower.search_borrowed_books("The Great Gatsby")
```
阅读全文
相关推荐
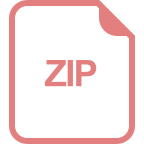
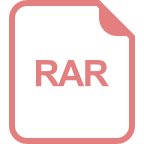
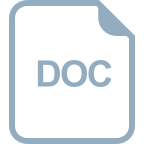














