检查一下这段代码的错误:struct node { char name[20]; int score; struct node *next;};void add_node(struct node **head, char *name, int score) { struct node *new_node = malloc(sizeof(struct node)); strcpy(new_node->name, name); new_node->score = score; new_node->next = NULL; if (*head == NULL) { *head = new_node; } else { struct node *tmp = *head; while (tmp->next != NULL) { tmp = tmp->next; } tmp->next = new_node; }}void add_flag(struct node **head) { struct node *new_node = malloc(sizeof(struct node)); strcpy(new_node->name, "flag"); new_node->score = -1; new_node->next = NULL; if (*head == NULL) { *head = new_node; } else { struct node *tmp = *head; while (tmp->next != NULL) { tmp = tmp->next; } tmp->next = new_node; }}
时间: 2024-04-02 11:32:47 浏览: 23
这段代码没有明显的语法错误,但是有一些潜在的问题需要注意:
1. 在使用 `malloc` 分配内存之后,需要检查是否分配成功。如果分配失败,返回的指针为 NULL,需要进行错误处理。
2. 在使用 `strcpy` 函数拷贝字符串之前,需要确保目标数组有足够的空间来存储源字符串。否则,会导致内存越界,引发未定义行为。
3. 在链表的操作中,需要注意链表头指针的变化。如果在函数中修改链表头指针的值,需要使用指向指针的指针(即 `struct node **head`)来传递链表头指针,以确保修改的值能够被调用者正确地获取。
另外,这段代码中的 `add_flag` 函数似乎没有实际作用,因为它只是在链表的末尾添加了一个特殊节点,但是没有给出该节点的用途。如果没有必要添加这个节点,可以考虑删除该函数。
相关问题
struct Student { int class_num; char id[15]; char name[10]; float score[3]; float sum; float course_av[3]; float course_pass[3]; float course_max[3]; float course_min[3]; }; //链表节点定义 struct Node { struct Student student; struct Node* next; }; //链表定义 struct List { struct Node* head; //链表头节点 int size; //链表长度 }; struct List* createList(); //创建链表 void destoryList(struct List* list); //销毁链表 struct Node* createNode(struct Student student); //创建节点 struct Node* getLastNode(struct List* list); //获取链表最后一个节点 struct Node* getBeforeNode(struct List* list, struct Student student); //获取上一个节点 void addList(struct List* list, struct Node* node); //将节点添加到链表中 #endif 这是个什么数据结构?看起来不像单链表
这是一个单链表的实现,每个链表节点包含一个 `struct Student` 类型的数据,以及一个指向下一个节点的指针 `next`。链表的头节点定义在 `struct List` 中的 `head` 中,链表的长度定义在 `struct List` 中的 `size` 中。函数包括创建链表、销毁链表、创建节点、获取最后一个节点、获取上一个节点、将节点添加到链表中等操作。
写一个程序,定义以下结构类型,调用函数创建一个动态链表,并且输出该链表。 struct Student { int num; char name[20]; float score[3]; struct Student * next; }; 测试用例:程序执行,提示请用户输入学生数据, 用户输入: 1, zhang, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 2, li, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 3, wang, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 0, 0, 0,0,0回车, 程序输出: 1, zhang, 70.00, 80.00, 90.00, 2, li, 70.00, 80.00, 90.00, 3, wang, 70.00, 80.00, 90.00
以下是一个示例程序,实现了上述功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
int num;
char name[20];
float score[3];
struct Student *next;
};
// 创建一个新结点
struct Student *create_node(int num, char *name, float *score) {
struct Student *new_node = (struct Student*)malloc(sizeof(struct Student));
new_node->num = num;
strcpy(new_node->name, name);
for (int i = 0; i < 3; i++) {
new_node->score[i] = score[i];
}
new_node->next = NULL;
return new_node;
}
// 在链表末尾添加一个新结点
void add_node(struct Student *head, struct Student *new_node) {
struct Student *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_node;
}
// 输出链表中的所有结点
void print_list(struct Student *head) {
struct Student *p = head;
while (p != NULL) {
printf("%d, %s, %.2f, %.2f, %.2f\n", p->num, p->name, p->score[0], p->score[1], p->score[2]);
p = p->next;
}
}
int main() {
// 创建链表头结点
struct Student *head = (struct Student*)malloc(sizeof(struct Student));
head->num = 0;
strcpy(head->name, "head");
head->next = NULL;
// 读入学生数据,并创建结点
while (1) {
int num;
char name[20];
float score[3];
printf("请输入学生数据(格式: 学号 姓名 成绩1 成绩2 成绩3): ");
scanf("%d%s%f%f%f", &num, name, &score[0], &score[1], &score[2]);
if (num == 0) {
break;
}
// 创建新结点
struct Student *new_node = create_node(num, name, score);
// 将新结点添加到链表末尾
相关推荐
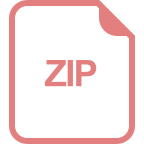
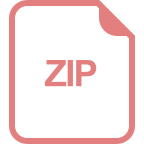
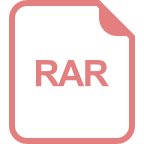












