如何利用pytorch编写softmax层
时间: 2023-06-03 12:01:57 浏览: 202
为了利用PyTorch编写softmax层,您可以按照以下代码示例进行操作:
``` python
import torch.nn as nn
class SoftmaxLayer(nn.Module):
def __init__(self, input_size, output_size):
super(SoftmaxLayer, self).__init__()
self.linear = nn.Linear(input_size, output_size)
def forward(self, x):
x = self.linear(x)
return nn.functional.softmax(x, dim=1)
```
在此示例中,我们定义了一个名为“SoftmaxLayer”的新类,以便我们可以将其作为我们神经网络的一层。我们在此类中实现了我们的softmax层,其中我们使用“nn.Linear”函数定义了一个线性层,并且在“forward”函数中使用“nn.functional.softmax”将线性输出转换为softmax输出。
您可以使用此类,将其添加到您的PyTorch神经网络中,以获得所需的softmax输出。
相关问题
利用pytorch编写图卷积神经网络代码
在PyTorch中,我们可以利用`torch_geometric`库来编写图卷积神经网络(Graph Convolutional Network, GCN)。以下是一个简单的例子,展示了如何创建一个基本的GCN模型:
```python
import torch
from torch_geometric.nn import GCNConv
class GraphConvNet(torch.nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GraphConvNet, self).__init__()
# 创建第一个GCN层
self.conv1 = GCNConv(in_channels, hidden_channels)
# 创建第二个GCN层
self.conv2 = GCNConv(hidden_channels, out_channels)
def forward(self, x, edge_index):
"""
x: 输入节点特征 (batch_size, num_nodes, in_channels)
edge_index: 边的连接数组 (2, num_edges) 或者 (num_nodes, num_nodes) 如果是无向图
"""
x = F.relu(self.conv1(x, edge_index)) # 应用ReLU激活函数
x = F.dropout(x, training=self.training) # 随机失活
x = self.conv2(x, edge_index) # 再次应用GCN层
return F.log_softmax(x, dim=1) # 输出节点分类的概率分布
# 使用示例
model = GraphConvNet(64, 16, 7) # 假设我们有64维的输入特征,16作为隐藏通道数,7类作为输出类别
x = torch.randn(100, 64) # 100个样本,每个样本64维特征
edge_index = torch.LongTensor([[0, 1, 2, 3], [1, 2, 3, 0]]) # 示例边的连接数组,如果是无向图则形状应为(num_nodes, num_nodes)
out = model(x, edge_index)
```
在这个例子中,`GCNConv`是核心模块,它接收输入特征`x`和边的连接数组`edge_index`,然后通过多次传递信息并更新节点特征来进行图卷积操作。
使用pytorch编写决策树代码
非常感谢您的提问。以下是使用 PyTorch 编写决策树的代码示例:
```python
import torch
import torch.nn as nn
class DecisionTree(nn.Module):
def __init__(self, input_dim, output_dim):
super(DecisionTree, self).__init__()
self.fc1 = nn.Linear(input_dim, 64)
self.fc2 = nn.Linear(64, 32)
self.fc3 = nn.Linear(32, output_dim)
self.relu = nn.ReLU()
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.relu(x)
x = self.fc3(x)
x = self.softmax(x)
return x
```
希望这个代码示例能够帮助您!
阅读全文
相关推荐
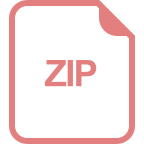
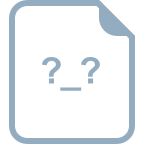
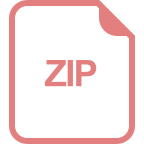


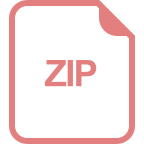
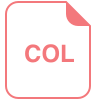








