编写一段C++代码,要求设计二维数组矩阵类模版,然后用成员函数实现矩阵加法,用友元函数实现矩阵减法,同时矩阵的输入输出要用到文件的输入输出管理
时间: 2024-03-25 16:41:40 浏览: 15
以下是符合题目要求的 C++ 代码示例:
```c++
#include <iostream>
#include <fstream>
using namespace std;
template <class T>
class Matrix {
private:
int rows, cols;
T **data;
public:
Matrix(int r, int c) : rows(r), cols(c) {
data = new T*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new T[cols];
}
}
Matrix(const Matrix<T> &mat) : rows(mat.rows), cols(mat.cols) {
data = new T*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new T[cols];
for (int j = 0; j < cols; j++) {
data[i][j] = mat.data[i][j];
}
}
}
~Matrix() {
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
void input(const char *filename) {
ifstream fin(filename);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
fin >> data[i][j];
}
}
fin.close();
}
void output(const char *filename) const {
ofstream fout(filename);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
fout << data[i][j] << " ";
}
fout << endl;
}
fout.close();
}
Matrix<T> operator+(const Matrix<T> &mat) const {
Matrix<T> result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] + mat.data[i][j];
}
}
return result;
}
template <typename U>
friend Matrix<U> operator-(const Matrix<U> &mat1, const Matrix<U> &mat2);
};
template <typename U>
Matrix<U> operator-(const Matrix<U> &mat1, const Matrix<U> &mat2) {
Matrix<U> result(mat1.rows, mat1.cols);
for (int i = 0; i < mat1.rows; i++) {
for (int j = 0; j < mat1.cols; j++) {
result.data[i][j] = mat1.data[i][j] - mat2.data[i][j];
}
}
return result;
}
int main() {
Matrix<int> mat1(2, 2);
mat1.input("mat1.txt");
Matrix<int> mat2(mat1);
Matrix<int> mat3(2, 2);
mat3.input("mat3.txt");
Matrix<int> mat4 = mat1 + mat2;
Matrix<int> mat5 = mat3 - mat4;
mat5.output("mat5.txt");
return 0;
}
```
在本示例中,我们设计了一个二维数组矩阵类模版 `Matrix`,具有行数、列数和数据成员 `data`。其中,构造函数和析构函数分别用于分配和释放内存,`input` 和 `output` 成员函数分别用于从文件中读取矩阵和将矩阵输出到文件。重载了 `+` 运算符用于实现矩阵加法,而 `operator-` 则是一个友元函数,用于实现矩阵减法。
在 `main` 函数中,我们首先创建了一个 `2 x 2` 的矩阵 `mat1`,并从文件中读取了它的值。然后,我们创建了一个与 `mat1` 相同的矩阵 `mat2`,并将其初始化为 `mat1`。接着,我们创建了另一个 `2 x 2` 的矩阵 `mat3`,并从文件中读取了它的值。最后,我们将 `mat1` 和 `mat2` 相加,将结果存储在 `mat4` 中,然后将 `mat3` 减去 `mat4`,将结果存储在 `mat5` 中,并将 `mat5` 输出到文件中。
请注意,本示例中的文件输入输出实现方式仅供参考。在实际应用中,应该根据实际情况进行修改和优化。
相关推荐
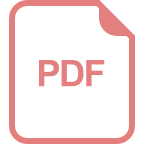
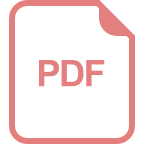













