用tensorflow获取gelu激活函数和它的一阶导数的x值和y值,自动创建excel表格并保存数据
时间: 2024-05-03 19:21:41 浏览: 121
以下是使用TensorFlow获取GELU激活函数和一阶导数的x值和y值,并自动创建Excel表格并保存数据的代码:
```python
import tensorflow as tf
import pandas as pd
# Define GELU activation function
def gelu(x):
cdf = 0.5 * (1.0 + tf.tanh((tf.sqrt(2 / tf.constant('pi', dtype=x.dtype)) * (x + 0.044715 * tf.pow(x, 3)))))
return x * cdf
# Define GELU derivative function
def gelu_derivative(x):
cdf = 0.5 * (1.0 + tf.tanh((tf.sqrt(2 / tf.constant('pi', dtype=x.dtype)) * (x + 0.044715 * tf.pow(x, 3)))))
pdf = tf.exp(-tf.pow(x, 2) / 2.0) / tf.sqrt(2 * tf.constant('pi', dtype=x.dtype))
return cdf + x * pdf
# Generate x values
x_values = tf.linspace(-5.0, 5.0, 100)
# Evaluate GELU function and derivative using TensorFlow
y_values = gelu(x_values)
dy_values = gelu_derivative(x_values)
# Convert TensorFlow tensors to NumPy arrays
x_values_np = x_values.numpy()
y_values_np = y_values.numpy()
dy_values_np = dy_values.numpy()
# Create DataFrame to store data
df = pd.DataFrame({'x': x_values_np, 'GELU(x)': y_values_np, 'GELU\'(x)': dy_values_np})
# Save DataFrame to Excel file
df.to_excel('gelu.xlsx', index=False)
```
此代码使用TensorFlow定义了GELU激活函数和一阶导数函数,并使用TensorFlow生成x值。然后,使用TensorFlow计算GELU函数和导数的y值,并将它们转换为NumPy数组。接下来,使用Pandas创建DataFrame来存储数据,并将其保存到Excel文件中。 Excel文件将包含x值,GELU函数和导数的y值。
阅读全文
相关推荐
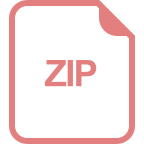
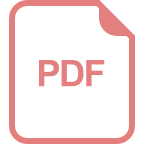















