在循环队列中,以front和length分别表示循环队列中的队头位置和队列中所含元素的个数。试完成循环队列判断队空、判断队满入队和出队函数、获取队头元素及输出的操作,在主函数中调用以上函数,完成杨辉三角的输出。 【希冀测试数据说明】 1)从键盘输入数字n 2)输出对应的杨辉三角;若队列满了,输出杨辉三角的前面若干行;然后输出队列已满;
时间: 2023-05-28 09:08:15 浏览: 127
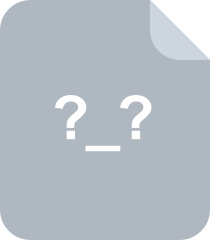
循环队列实现杨辉三角的输出

#include <stdio.h>
#define MAXSIZE 100 // 定义循环队列的最大长度为100
int queue[MAXSIZE]; // 循环队列数组
int front = 0, length = 0; // 队头位置和队列元素个数
// 判断队列是否为空
int is_empty() {
return length == 0;
}
// 判断队列是否已满
int is_full() {
return length == MAXSIZE;
}
// 入队
void enqueue(int x) {
if (is_full()) {
printf("队列已满\n");
return;
}
queue[(front + length) % MAXSIZE] = x; // 计算插入位置并插入元素
length++; // 队列元素个数加1
}
// 出队
int dequeue() {
if (is_empty()) {
printf("队列为空\n");
return -1;
}
int x = queue[front]; // 取出队头元素
front = (front + 1) % MAXSIZE; // 计算队头位置
length--; // 队列元素个数减1
return x;
}
// 获取队头元素
int get_front() {
if (is_empty()) {
printf("队列为空\n");
return -1;
}
return queue[front];
}
// 输出队列中所有元素
void output() {
if (is_empty()) {
printf("队列为空\n");
return;
}
int i;
for (i = 0; i < length; i++) {
printf("%d ", queue[(front + i) % MAXSIZE]);
}
printf("\n");
}
// 输出杨辉三角
void yanghui_triangle(int n) {
int i, j;
for (i = 1; i <= n; i++) {
for (j = 1; j <= i; j++) {
if (j == 1 || j == i) {
enqueue(1); // 第一列和对角线上的元素为1
} else {
enqueue(get_front() + queue[(front + 1) % MAXSIZE]); // 计算当前位置的元素
dequeue(); // 弹出队头元素
}
printf("%d ", get_front()); // 输出当前位置的元素
}
printf("\n");
}
}
int main() {
int n;
printf("请输入数字n:");
scanf("%d", &n);
yanghui_triangle(n);
return 0;
}
阅读全文
相关推荐
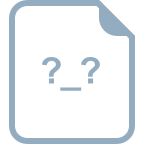














