android MediaCodec 录屏时长和大小功能实现完整代码
时间: 2024-02-01 18:14:33 浏览: 23
以下是一个简单的 Android MediaCodec 录屏实现的完整代码示例,可以记录屏幕并保存为 MP4 文件。
```
public class ScreenRecorder {
private static final String TAG = "ScreenRecorder";
private static final String MIME_TYPE = "video/avc";
private static final int FRAME_RATE = 30; // 帧率
private static final int I_FRAME_INTERVAL = 1; // I 帧间隔
private static final int BIT_RATE = 6000000; // 比特率
private static final int REQUEST_CODE = 1;
private static int width;
private static int height;
private static int dpi;
private MediaProjection mediaProjection;
private MediaCodec mediaCodec;
private MediaMuxer mediaMuxer;
private Surface surface;
private VirtualDisplay virtualDisplay;
private boolean isRecording;
private String outputFilePath;
private Context context;
public ScreenRecorder(Context context) {
this.context = context;
}
public void startRecording(int resultCode, Intent data) {
if (isRecording) {
Log.e(TAG, "Already recording");
return;
}
mediaProjection = createMediaProjection(resultCode, data);
if (mediaProjection == null) {
Log.e(TAG, "MediaProjection is null");
return;
}
width = context.getResources().getDisplayMetrics().widthPixels;
height = context.getResources().getDisplayMetrics().heightPixels;
dpi = context.getResources().getDisplayMetrics().densityDpi;
mediaCodec = createMediaCodec();
mediaMuxer = createMediaMuxer();
mediaCodec.start();
virtualDisplay = createVirtualDisplay();
isRecording = true;
}
public void stopRecording() {
if (!isRecording) {
Log.e(TAG, "Not recording");
return;
}
isRecording = false;
if (virtualDisplay != null) {
virtualDisplay.release();
virtualDisplay = null;
}
if (mediaCodec != null) {
mediaCodec.stop();
mediaCodec.release();
mediaCodec = null;
}
if (mediaProjection != null) {
mediaProjection.stop();
mediaProjection = null;
}
if (mediaMuxer != null) {
mediaMuxer.stop();
mediaMuxer.release();
mediaMuxer = null;
}
}
private MediaProjection createMediaProjection(int resultCode, Intent data) {
return ((MediaProjectionManager) context.getSystemService(Context.MEDIA_PROJECTION_SERVICE))
.getMediaProjection(resultCode, data);
}
private MediaCodec createMediaCodec() {
MediaFormat format = MediaFormat.createVideoFormat(MIME_TYPE, width, height);
format.setInteger(MediaFormat.KEY_COLOR_FORMAT, MediaCodecInfo.CodecCapabilities.COLOR_FormatSurface);
format.setInteger(MediaFormat.KEY_BIT_RATE, BIT_RATE);
format.setInteger(MediaFormat.KEY_FRAME_RATE, FRAME_RATE);
format.setInteger(MediaFormat.KEY_I_FRAME_INTERVAL, I_FRAME_INTERVAL);
MediaCodec codec = null;
try {
codec = MediaCodec.createEncoderByType(MIME_TYPE);
codec.configure(format, null, null, MediaCodec.CONFIGURE_FLAG_ENCODE);
surface = codec.createInputSurface();
} catch (IOException e) {
e.printStackTrace();
}
return codec;
}
private MediaMuxer createMediaMuxer() {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss", Locale.US);
String dateTime = dateFormat.format(new Date());
String fileName = "screen_record_" + dateTime + ".mp4";
outputFilePath = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_MOVIES)
+ File.separator + fileName;
MediaMuxer muxer = null;
try {
muxer = new MediaMuxer(outputFilePath, MediaMuxer.OutputFormat.MUXER_OUTPUT_MPEG_4);
} catch (IOException e) {
e.printStackTrace();
}
return muxer;
}
private VirtualDisplay createVirtualDisplay() {
return mediaProjection.createVirtualDisplay(TAG, width, height, dpi,
DisplayManager.VIRTUAL_DISPLAY_FLAG_PUBLIC, surface, null, null);
}
public boolean isRecording() {
return isRecording;
}
public String getOutputFilePath() {
return outputFilePath;
}
}
```
使用该类,你只需要在你的 Activity 中调用 startRecording() 方法来开始录制,调用 stopRecording() 方法来停止录制。录制完成后,你可以通过调用 getOutputFilePath() 方法来获得输出文件的路径。
以下是一个简单的使用示例:
```
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_CODE = 1;
private ScreenRecorder screenRecorder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
screenRecorder = new ScreenRecorder(this);
findViewById(R.id.start_button).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startRecording();
}
});
findViewById(R.id.stop_button).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
stopRecording();
}
});
}
private void startRecording() {
Intent mediaProjectionIntent = ((MediaProjectionManager) getSystemService(Context.MEDIA_PROJECTION_SERVICE))
.createScreenCaptureIntent();
startActivityForResult(mediaProjectionIntent, REQUEST_CODE);
}
private void stopRecording() {
screenRecorder.stopRecording();
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_CODE && resultCode == RESULT_OK && data != null) {
screenRecorder.startRecording(resultCode, data);
}
}
}
```
补充说明:该代码仅仅是一个简单的示例,可能存在许多潜在问题和优化空间。请在实际使用中自行测试和改进。
相关推荐
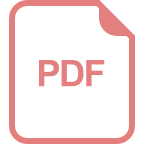
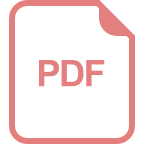
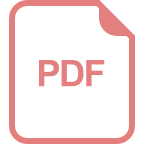














