公司现有以下设备:普通电视机、DVD、带DVD的电视机,带DVD的电视机的售价为普通电视机和DVD单价之和的80%。请编制-程序要求对所有的库存设备都能实现按照品名进行显示、修改、查找、增加、删除、保存的功能。用c语言编程,不使用链表和指针。
时间: 2023-07-10 22:14:02 浏览: 169
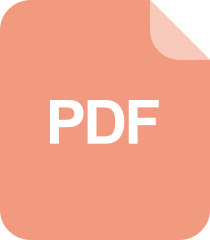
通信与网络中的基于T-DMB标准的手机电视解决方案
好的,我可以为你编写这个程序。由于不使用链表和指针,我会使用数组来存储设备信息。以下是程序的基本框架:
```c
#include <stdio.h>
#include <string.h>
// 定义设备结构体
struct Device {
char name[50]; // 设备名称
int type; // 设备类型,0表示普通电视机,1表示DVD,2表示带DVD的电视机
float price; // 设备价格
};
// 定义函数声明
void showAllDevices(struct Device devices[], int count);
void modifyDevice(struct Device devices[], int count);
void searchDevice(struct Device devices[], int count);
void addDevice(struct Device devices[], int *count);
void deleteDevice(struct Device devices[], int *count);
void saveDevices(struct Device devices[], int count);
int main() {
struct Device devices[100]; // 定义设备数组
int count = 0; // 记录设备数量
// 读取已保存的设备信息
FILE *fp = fopen("devices.txt", "r");
if (fp) {
while (fscanf(fp, "%s %d %f", devices[count].name, &devices[count].type, &devices[count].price) != EOF) {
count++;
}
fclose(fp);
}
// 显示菜单
int choice;
do {
printf("1. 显示所有设备\n");
printf("2. 修改设备信息\n");
printf("3. 查找设备\n");
printf("4. 增加设备\n");
printf("5. 删除设备\n");
printf("6. 保存设备信息\n");
printf("0. 退出程序\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
showAllDevices(devices, count);
break;
case 2:
modifyDevice(devices, count);
break;
case 3:
searchDevice(devices, count);
break;
case 4:
addDevice(devices, &count);
break;
case 5:
deleteDevice(devices, &count);
break;
case 6:
saveDevices(devices, count);
break;
case 0:
printf("谢谢使用!\n");
break;
default:
printf("无效操作,请重新选择!\n");
break;
}
} while (choice != 0);
return 0;
}
// 显示所有设备
void showAllDevices(struct Device devices[], int count) {
printf("设备名称\t设备类型\t设备价格\n");
for (int i = 0; i < count; i++) {
printf("%s\t\t", devices[i].name);
switch (devices[i].type) {
case 0:
printf("普通电视机\t");
break;
case 1:
printf("DVD\t\t");
break;
case 2:
printf("带DVD的电视机\t");
break;
}
printf("%.2f\n", devices[i].price);
}
}
// 修改设备信息
void modifyDevice(struct Device devices[], int count) {
char name[50];
printf("请输入要修改的设备名称:");
scanf("%s", name);
int found = 0;
for (int i = 0; i < count; i++) {
if (strcmp(devices[i].name, name) == 0) {
printf("请输入新的设备类型(0表示普通电视机,1表示DVD,2表示带DVD的电视机):");
scanf("%d", &devices[i].type);
printf("请输入新的设备价格:");
scanf("%f", &devices[i].price);
printf("修改成功!\n");
found = 1;
break;
}
}
if (!found) {
printf("设备不存在!\n");
}
}
// 查找设备
void searchDevice(struct Device devices[], int count) {
char name[50];
printf("请输入要查找的设备名称:");
scanf("%s", name);
int found = 0;
for (int i = 0; i < count; i++) {
if (strcmp(devices[i].name, name) == 0) {
printf("设备名称\t设备类型\t设备价格\n");
printf("%s\t\t", devices[i].name);
switch (devices[i].type) {
case 0:
printf("普通电视机\t");
break;
case 1:
printf("DVD\t\t");
break;
case 2:
printf("带DVD的电视机\t");
break;
}
printf("%.2f\n", devices[i].price);
found = 1;
break;
}
}
if (!found) {
printf("设备不存在!\n");
}
}
// 增加设备
void addDevice(struct Device devices[], int *count) {
printf("请输入设备名称:");
scanf("%s", devices[*count].name);
printf("请输入设备类型(0表示普通电视机,1表示DVD,2表示带DVD的电视机):");
scanf("%d", &devices[*count].type);
printf("请输入设备价格:");
scanf("%f", &devices[*count].price);
(*count)++;
printf("添加成功!\n");
}
// 删除设备
void deleteDevice(struct Device devices[], int *count) {
char name[50];
printf("请输入要删除的设备名称:");
scanf("%s", name);
int found = 0;
for (int i = 0; i < *count; i++) {
if (strcmp(devices[i].name, name) == 0) {
for (int j = i; j < *count - 1; j++) {
devices[j] = devices[j + 1];
}
(*count)--;
printf("删除成功!\n");
found = 1;
break;
}
}
if (!found) {
printf("设备不存在!\n");
}
}
// 保存设备信息
void saveDevices(struct Device devices[], int count) {
FILE *fp = fopen("devices.txt", "w");
for (int i = 0; i < count; i++) {
fprintf(fp, "%s %d %.2f\n", devices[i].name, devices[i].type, devices[i].price);
}
fclose(fp);
printf("保存成功!\n");
}
```
以上程序中,我定义了一个 `Device` 结构体,用来存储设备的名称、类型和价格。我使用了一个长度为 100 的数组来存储设备信息,数组的下标从 0 开始。程序提供了显示所有设备、修改设备信息、查找设备、增加设备、删除设备、保存设备信息等功能。程序运行时,会从文件中读取已保存的设备信息,程序退出时会将设备信息保存到文件中。
阅读全文
相关推荐
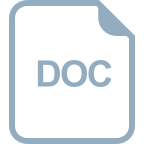
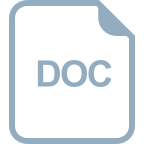







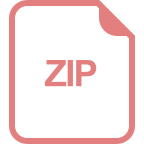
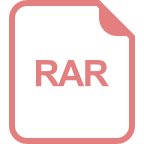
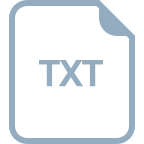
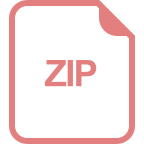
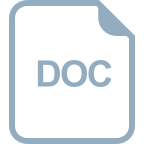



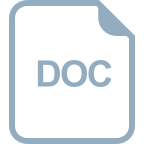