k210识别数字后给stm32f103rct6传递参数的代码
时间: 2024-06-09 19:06:34 浏览: 115
假设你使用的是K210的Maixduino开发板,以下是一个简单的示例代码,用于将数字识别结果通过串口传递给STM32F103RCT6:
```c
#include <Maixduino.h>
#include <stdio.h>
// 定义串口对象
HardwareSerial Serial(1);
void setup() {
// 初始化串口
Serial.begin(115200);
while(!Serial) delay(10);
// 初始化KPU
if(kpu_load_kmodel(&task, model_data) != 0) {
printf("Load kmodel failed\n");
while(1);
}
if(kpu_init(&task, 0) != 0) {
printf("Init kpu failed\n");
while(1);
}
}
void loop() {
// 获取摄像头图像
camera_fb_t *fb = camera_fb_get();
if(!fb) {
printf("Camera capture failed\n");
return;
}
// 运行KPU模型
uint8_t *image_data = fb->buf;
if(kpu_run_kmodel(&task, image_data, out_buf, NULL) != 0) {
printf("Run kpu failed\n");
return;
}
// 识别结果处理
float *output_data = (float *)out_buf;
int predict = 0;
float max_prob = 0;
for(int i = 0; i < OUTPUT_NUM; i++) {
float prob = output_data[i];
if(prob > max_prob) {
max_prob = prob;
predict = i;
}
}
// 将识别结果通过串口发送给STM32
Serial.printf("Recognized digit: %d\n", predict);
Serial.flush();
// 释放摄像头图像
camera_fb_return(fb);
}
```
在STM32F103RCT6中,你需要编写代码来接收串口数据,并根据需要进行处理。以下是一个简单的示例代码,用于接收来自K210的数字识别结果:
```c
#include "stm32f10x.h"
#include <stdio.h>
// 定义串口对象
USART_InitTypeDef USART_InitStructure;
void USART_Configuration(void) {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
int main(void) {
// 初始化串口
USART_Configuration();
while (1) {
// 接收串口数据
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
uint8_t c = USART_ReceiveData(USART1);
// 处理数字识别结果
if(c >= '0' && c <= '9') {
printf("Received digit: %c\n", c);
}
}
}
```
请注意,以上代码仅为示例代码,你需要根据自己的具体情况进行修改。
阅读全文
相关推荐
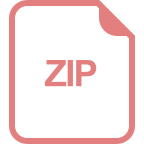
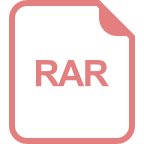

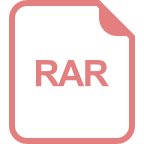
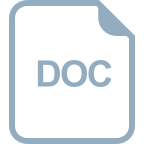
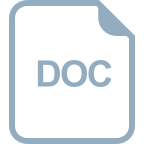











