用C++语言实现前缀表达式求值
时间: 2023-09-15 10:21:00 浏览: 128
这里是使用C语言实现前缀表达式求值的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
void push(int value) {
if (top >= MAX_SIZE - 1) {
printf("Stack overflow");
exit(EXIT_FAILURE);
}
stack[++top] = value;
}
int pop() {
if (top == -1) {
printf("Stack underflow");
exit(EXIT_FAILURE);
}
return stack[top--];
}
int evaluate(char* expression) {
int i = 0, operand1, operand2;
char ch;
while ((ch = expression[i++]) != '\0') {
if (ch >= '0' && ch <= '9') {
push(ch - '0');
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
operand2 = pop();
operand1 = pop();
switch (ch) {
case '+':
push(operand1 + operand2);
break;
case '-':
push(operand1 - operand2);
break;
case '*':
push(operand1 * operand2);
break;
case '/':
push(operand1 / operand2);
break;
}
}
}
return pop();
}
int main() {
char expression[MAX_SIZE];
printf("Enter the prefix expression: ");
scanf("%s", expression);
int result = evaluate(expression);
printf("Result: %d", result);
return 0;
}
```
该程序首先定义了一个栈来存储操作数。栈的push和pop操作用于在栈中添加和删除元素。evaluate函数用于评估前缀表达式。在该函数中,使用循环遍历表达式中的每个字符。如果字符是一个数字,则将其转换为整数并将其推送到栈中。如果字符是一个运算符,则从栈中弹出两个操作数,并使用该运算符对它们进行操作。最后,该函数返回栈中的最后一个元素,即表达式的结果。在main函数中,用户输入前缀表达式,然后调用evaluate函数来计算表达式并输出结果。
阅读全文
相关推荐



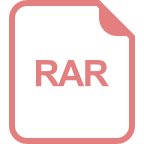














