c++代码的Float /time/ 意思
时间: 2023-10-31 22:18:57 浏览: 35
`Float /time/` 不是 C++ 代码的语法,可能是出现在某个文档或注释中的术语。一般来说,`float` 是 C++ 的一种数据类型,表示单精度浮点数,可以用来存储小数。而 `/time/` 可能是指时间相关的内容,但具体含义需要根据上下文和语境来确定。如果您能提供更多的上下文信息,我可以更好地帮助您解答。
相关问题
C/c++获取网络使用率
要获取网络使用率,可以通过以下步骤使用C/C++编写程序:
1. 使用系统函数获取网络流量信息,例如在Windows平台上,可以使用GetIfTable和GetIfEntry函数获取网络接口信息和流量统计数据。
2. 计算网络使用率,可以使用流量统计数据计算网络使用率,通常使用以下公式:使用率 = (接收字节数 + 发送字节数) / 带宽 * 时间间隔。
3. 可以使用定时器或循环方式来周期性地获取和计算网络使用率。
以下是一个简单的C++示例代码,可以获取并打印出当前网络使用率:
```c++
#include <iostream>
#include <windows.h>
#include <iphlpapi.h>
#pragma comment(lib, "iphlpapi.lib")
int main()
{
MIB_IFTABLE* ifTable = nullptr;
DWORD ifTableSize = 0;
// 获取网络接口信息
GetIfTable(ifTable, &ifTableSize, FALSE);
if (ifTableSize == 0)
{
std::cerr << "Failed to get interface table!" << std::endl;
return -1;
}
ifTable = (MIB_IFTABLE*)new char[ifTableSize];
if (GetIfTable(ifTable, &ifTableSize, FALSE) != NO_ERROR)
{
std::cerr << "Failed to get interface table!" << std::endl;
delete[] ifTable;
return -1;
}
// 计算网络使用率
ULONG totalBytes = 0;
ULONG totalBandwidth = 0;
for (int i = 0; i < ifTable->dwNumEntries; ++i)
{
MIB_IFROW& ifRow = ifTable->table[i];
if (ifRow.dwType == MIB_IF_TYPE_ETHERNET)
{
totalBytes += ifRow.dwInOctets + ifRow.dwOutOctets;
totalBandwidth += ifRow.dwSpeed;
}
}
static ULONG lastTotalBytes = 0;
static ULONG lastTime = GetTickCount();
ULONG currentTime = GetTickCount();
ULONG elapsedTime = (currentTime - lastTime) / 1000;
ULONG currentBytes = totalBytes - lastTotalBytes;
lastTotalBytes = totalBytes;
lastTime = currentTime;
float usage = (float)currentBytes / totalBandwidth * 8 / elapsedTime;
std::cout << "Network usage: " << usage << " Mbps" << std::endl;
delete[] ifTable;
return 0;
}
```
需要注意的是,代码中仅仅统计了Ethernet接口的流量信息,并且只计算了单向流量,实际应用中可能需要根据具体需求进行修改。
srtf最短剩余时间c++代码
下面是 SRTF (最短剩余时间优先) 调度算法的 C++ 代码示例:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct Process {
int pid; // 进程 ID
int at; // 到达时间
int bt; // 运行时间
int rt; // 剩余运行时间
};
struct Compare {
bool operator()(const Process& a, const Process& b) {
return a.rt > b.rt; // 按照剩余时间从小到大排序
}
};
void srtf(Process processes[], int n) {
priority_queue<Process, vector<Process>, Compare> pq;
int current_time = 0;
int completed = 0;
int waiting_time = 0;
int turnaround_time = 0;
pq.push(processes[0]);
int i = 1;
while (completed < n) {
if (pq.empty()) {
pq.push(processes[i++]);
continue;
}
Process p = pq.top();
pq.pop();
if (p.rt == p.bt) { // 第一次被调度
waiting_time += current_time - p.at;
} else {
waiting_time += current_time - p.rt - p.at;
}
turnaround_time += current_time - p.at + p.bt - p.rt;
current_time += p.rt;
while (i < n && current_time >= processes[i].at) {
pq.push(processes[i++]);
}
completed++;
p.rt = 0;
pq.push(p);
}
cout << "Average waiting time = " << (float)waiting_time / n << endl;
cout << "Average turnaround time = " << (float)turnaround_time / n << endl;
}
int main() {
Process processes[] = {
{1, 0, 5, 5},
{2, 1, 3, 3},
{3, 2, 8, 8},
{4, 3, 6, 6},
{5, 4, 4, 4},
};
int n = sizeof(processes) / sizeof(Process);
srtf(processes, n);
return 0;
}
```
相关推荐
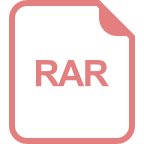
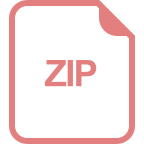
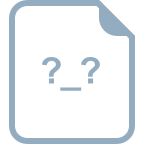












