springboot Thymeleaf增删改查
时间: 2023-07-09 11:47:51 浏览: 52
好的,我可以为您提供一些关于Spring Boot和Thymeleaf的基本增删改查操作的示例代码,如下所示:
1. 实体类定义
```
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// 省略setter和getter方法
}
```
2. 数据访问层
```
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
3. 服务层
```
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> findAll() {
return userRepository.findAll();
}
public User findById(Long id) {
return userRepository.findById(id).orElse(null);
}
public void save(User user) {
userRepository.save(user);
}
public void deleteById(Long id) {
userRepository.deleteById(id);
}
}
```
4. 控制器层
```
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/")
public String index(Model model) {
List<User> userList = userService.findAll();
model.addAttribute("userList", userList);
return "index";
}
@GetMapping("/add")
public String add(Model model) {
model.addAttribute("user", new User());
return "form";
}
@GetMapping("/edit/{id}")
public String edit(@PathVariable Long id, Model model) {
User user = userService.findById(id);
model.addAttribute("user", user);
return "form";
}
@PostMapping("/save")
public String save(@ModelAttribute User user) {
userService.save(user);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable Long id) {
userService.deleteById(id);
return "redirect:/";
}
}
```
5. 页面模板
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring Boot Thymeleaf Demo</title>
</head>
<body>
<h2>User List</h2>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${userList}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.email}"></td>
<td>
<a th:href="@{/edit/{id}(id=${user.id})}">Edit</a>
<a th:href="@{/delete/{id}(id=${user.id})}">Delete</a>
</td>
</tr>
</tbody>
</table>
<br>
<a th:href="@{/add}">Add User</a>
</body>
</html>
```
6. 表单模板
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring Boot Thymeleaf Demo</title>
</head>
<body>
<h2>[[${user.id} == null ? 'Add' : 'Edit']] User</h2>
<form th:action="@{/save}" method="post">
<input type="hidden" th:field="*{id}">
<label for="name">Name:</label>
<input type="text" th:field="*{name}">
<br>
<label for="email">Email:</label>
<input type="email" th:field="*{email}">
<br>
<button type="submit">Save</button>
<a th:href="@{/}">Cancel</a>
</form>
</body>
</html>
```
以上是一个简单的Spring Boot和Thymeleaf的增删改查示例,可以根据自己的需要进行修改和扩展。
相关推荐
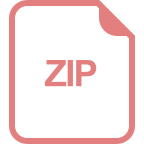














