用vue帮我写个贪吃蛇
时间: 2023-07-12 12:50:07 浏览: 81
好的,以下是一个简单的 Vue 实现的贪吃蛇游戏示例代码,供您参考:
```html
<template>
<div class="snake-game">
<div class="snake-board">
<div class="snake-grid" v-for="(row, rowIndex) in board" :key="rowIndex">
<div class="snake-cell" v-for="(cell, cellIndex) in row" :key="cellIndex" :class="{ 'snake': cell === 1, 'food': cell === 2 }"></div>
</div>
</div>
<div class="snake-score">Score: {{ score }}</div>
</div>
</template>
<script>
export default {
data() {
return {
board: [],
snake: [],
food: {},
direction: 'right',
score: 0,
intervalId: null
}
},
mounted() {
this.initGame();
},
methods: {
initGame() {
this.board = this.createBoard(20, 20);
this.snake = [
{ row: 10, col: 10 },
{ row: 10, col: 9 },
{ row: 10, col: 8 }
];
this.direction = 'right';
this.generateFood();
this.intervalId = setInterval(() => this.updateGame(), 100);
},
createBoard(rows, cols) {
const board = [];
for (let i = 0; i < rows; i++) {
const row = [];
for (let j = 0; j < cols; j++) {
row.push(0);
}
board.push(row);
}
return board;
},
generateFood() {
let row = Math.floor(Math.random() * this.board.length);
let col = Math.floor(Math.random() * this.board[0].length);
while (this.board[row][col] !== 0) {
row = Math.floor(Math.random() * this.board.length);
col = Math.floor(Math.random() * this.board[0].length);
}
this.food = { row, col };
this.board[row][col] = 2;
},
updateGame() {
const head = this.snake[0];
let newRow, newCol;
switch (this.direction) {
case 'up':
newRow = head.row - 1;
newCol = head.col;
break;
case 'down':
newRow = head.row + 1;
newCol = head.col;
break;
case 'left':
newRow = head.row;
newCol = head.col - 1;
break;
case 'right':
newRow = head.row;
newCol = head.col + 1;
break;
}
if (newRow < 0 || newRow >= this.board.length || newCol < 0 || newCol >= this.board[0].length || this.board[newRow][newCol] === 1) {
clearInterval(this.intervalId);
alert('Game Over!');
return;
}
this.snake.unshift({ row: newRow, col: newCol });
if (newRow === this.food.row && newCol === this.food.col) {
this.score++;
this.generateFood();
} else {
const tail = this.snake.pop();
this.board[tail.row][tail.col] = 0;
}
this.board[newRow][newCol] = 1;
},
handleKeyDown(event) {
switch (event.keyCode) {
case 37:
if (this.direction !== 'right') {
this.direction = 'left';
}
break;
case 38:
if (this.direction !== 'down') {
this.direction = 'up';
}
break;
case 39:
if (this.direction !== 'left') {
this.direction = 'right';
}
break;
case 40:
if (this.direction !== 'up') {
this.direction = 'down';
}
break;
}
}
},
created() {
document.addEventListener('keydown', this.handleKeyDown);
},
destroyed() {
document.removeEventListener('keydown', this.handleKeyDown);
}
};
</script>
<style scoped>
.snake-game {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
}
.snake-board {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 400px;
border: 1px solid black;
}
.snake-grid {
display: flex;
}
.snake-cell {
width: 20px;
height: 20px;
border: 1px solid lightgray;
}
.snake {
background-color: black;
}
.food {
background-color: red;
}
.snake-score {
margin-top: 10px;
font-size: 20px;
font-weight: bold;
}
</style>
```
在组件内,我们定义了以下数据和方法:
- `board`:二维数组,表示游戏面板,初始为全 0。
- `snake`:数组,表示蛇身体坐标。
- `food`:对象,表示食物坐标。
- `direction`:字符串,表示蛇的运动方向。
- `score`:整数,表示游戏得分。
- `intervalId`:定时器 ID,用于暂停游戏。
方法:
- `initGame()`:初始化游戏,设置初始值并开始游戏。
- `createBoard(rows, cols)`:生成指定行数和列数的游戏面板。
- `generateFood()`:随机生成食物坐标。
- `updateGame()`:更新游戏状态,包括蛇的位置、得分、食物等。
- `handleKeyDown(event)`:监听键盘事件,根据方向键调整蛇的运动方向。
在组件的 `mounted()` 钩子中调用 `initGame()` 方法,即可开始游戏。
注意,此示例代码只是一个简单的实现,并未考虑性能和代码优化等问题。实际开发中,需要根据具体需求进行改进和优化。
阅读全文
相关推荐
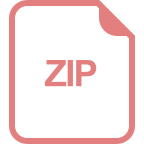
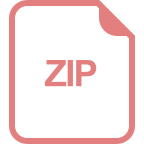
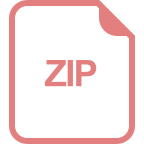















