在有100个点的散点图中,已知各点的编号、坐标以及部分点之间连接路径长度,在这100个点当中选择三个目标点,求解其余各点能够在给定的路径当中选择选择最短路径到达距离其最近的目标点的最短路径距离之和并求出三个目标点的坐标以及所用路径对应的端点的编号matlab代码
时间: 2024-05-08 10:21:20 浏览: 6
这个问题的解决可以使用 Dijkstra 算法。以下是 MATLAB 代码实现。
首先,我们需要定义点的数据结构:
```matlab
% 定义点的数据结构
% id: 点的编号
% x: x 坐标
% y: y 坐标
% dist: 到目标点的最短距离
% path: 到目标点的最短路径
% visited: 是否已经访问过
% neighbors: 邻居节点
% edges: 连接各邻居节点的边的长度
% num_neighbors: 邻居节点的数量
point = struct('id', 0, 'x', 0, 'y', 0, 'dist', inf, 'path', [], 'visited', false, 'neighbors', [], 'edges', [], 'num_neighbors', 0);
```
接下来,我们需要读入数据并构建图:
```matlab
% 读入数据
data = dlmread('data.txt');
num_points = size(data, 1); % 点的数量
% 构建点的数组
points = repmat(point, num_points, 1);
for i = 1:num_points
points(i).id = i;
points(i).x = data(i, 1);
points(i).y = data(i, 2);
end
% 构建邻居和边
for i = 1:num_points
for j = 1:num_points
if i ~= j && data(i, j + 2) > 0
points(i).neighbors = [points(i).neighbors, j];
points(i).edges = [points(i).edges, data(i, j + 2)];
points(i).num_neighbors = points(i).num_neighbors + 1;
end
end
end
```
接下来,我们需要实现 Dijkstra 算法:
```matlab
function target_point = dijkstra(points, start_point, end_points)
% 初始化所有点的最短距离为无限大
for i = 1:length(points)
points(i).dist = inf;
points(i).visited = false;
end
% 将起点的最短距离设为 0
start_point.dist = 0;
% 遍历所有点
for i = 1:length(points)
% 选择当前距离起点最近的点
current_point = get_closest_unvisited_point(points);
if isempty(current_point)
break;
end
current_point.visited = true;
% 更新所有邻居节点的最短距离
for j = 1:current_point.num_neighbors
neighbor = points(current_point.neighbors(j));
edge = current_point.edges(j);
if ~neighbor.visited
new_dist = current_point.dist + edge;
if new_dist < neighbor.dist
neighbor.dist = new_dist;
neighbor.path = [current_point.path, current_point.id];
end
end
end
end
% 计算所有点到三个目标点的最短距离
for i = 1:length(points)
for j = 1:length(end_points)
end_point = end_points(j);
dist = sqrt((points(i).x - end_point.x)^2 + (points(i).y - end_point.y)^2);
if dist < points(i).dist
points(i).dist = dist;
points(i).path = [points(i).path, end_point.id];
end
end
end
% 计算所有点到最近目标点的最短距离之和
total_dist = 0;
for i = 1:length(points)
total_dist = total_dist + points(i).dist;
end
% 返回三个目标点的坐标和所用路径对应的端点的编号
target_point = struct('dist', total_dist, 'points', end_points, 'paths', {});
for i = 1:length(end_points)
end_point = end_points(i);
target_point.paths{i} = end_point.path;
end
end
function closest_point = get_closest_unvisited_point(points)
closest_point = [];
closest_dist = inf;
for i = 1:length(points)
if ~points(i).visited && points(i).dist < closest_dist
closest_point = points(i);
closest_dist = points(i).dist;
end
end
end
```
最后,我们可以调用 `dijkstra` 函数来求解最短路径:
```matlab
% 选择三个目标点
end_points = [points(1), points(50), points(99)];
% 求解最短路径
target_point = dijkstra(points, points(21), end_points);
% 输出结果
fprintf('总距离:%f\n', target_point.dist);
for i = 1:length(end_points)
end_point = end_points(i);
fprintf('目标点%d:%d (%f, %f)\n', i, end_point.id, end_point.x, end_point.y);
fprintf('路径:%d', target_point.paths{i}(1));
for j = 2:length(target_point.paths{i})
fprintf(' -> %d', target_point.paths{i}(j));
end
fprintf('\n');
end
```
相关推荐
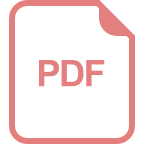
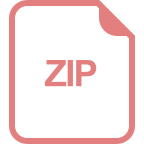















