使用flutter设计一个蓝牙控制界面,进入页面后,自动搜索显示蓝牙设备列表,搜搜索进度显示,有控制按钮,有通讯功能,支持空安全,请列出代码和依赖控件
时间: 2024-02-29 20:53:14 浏览: 20
好的,下面是一个简单的蓝牙控制界面的Flutter代码,包含了搜索蓝牙设备、连接设备、发送数据等功能。
依赖控件:
- flutter_blue: ^0.8.1
- cupertino_icons: ^1.0.2
```dart
import 'package:flutter/material.dart';
import 'package:flutter_blue/flutter_blue.dart';
class BluetoothControlPage extends StatefulWidget {
@override
_BluetoothControlPageState createState() => _BluetoothControlPageState();
}
class _BluetoothControlPageState extends State<BluetoothControlPage> {
FlutterBlue flutterBlue = FlutterBlue.instance;
List<BluetoothDevice> devicesList = [];
BluetoothDevice? selectedDevice;
BluetoothCharacteristic? characteristic;
bool isScanning = false;
bool isConnected = false;
bool isConnecting = false;
TextEditingController textEditingController = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Bluetooth Control"),
),
body: Column(
children: [
SizedBox(height: 16),
ElevatedButton(
onPressed: isScanning ? null : startScan,
child: Text(isScanning ? "Scanning..." : "Start Scan"),
),
SizedBox(height: 16),
Expanded(
child: ListView.builder(
itemCount: devicesList.length,
itemBuilder: (BuildContext context, int index) {
BluetoothDevice device = devicesList[index];
return ListTile(
title: Text(device.name),
subtitle: Text(device.id.toString()),
trailing: selectedDevice == device
? Icon(Icons.check, color: Colors.green)
: null,
onTap: () => selectDevice(device),
);
},
),
),
SizedBox(height: 16),
isConnected
? Column(
children: [
Text("Connected to ${selectedDevice?.name ?? ""}"),
SizedBox(height: 16),
TextField(
controller: textEditingController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: "Send Data",
),
),
SizedBox(height: 16),
ElevatedButton(
onPressed: send,
child: Text("Send"),
),
],
)
: SizedBox(),
],
),
);
}
void startScan() {
setState(() {
isScanning = true;
});
flutterBlue.scan().listen((scanResult) {
if (!devicesList.contains(scanResult.device)) {
setState(() {
devicesList.add(scanResult.device);
});
}
}).onDone(() {
setState(() {
isScanning = false;
});
});
}
void selectDevice(BluetoothDevice device) async {
setState(() {
isConnecting = true;
});
await device.connect();
List<BluetoothService> services = await device.discoverServices();
for (BluetoothService service in services) {
for (BluetoothCharacteristic characteristic in service.characteristics) {
if (characteristic.properties.read &&
characteristic.properties.write &&
characteristic.properties.notify) {
setState(() {
this.selectedDevice = device;
this.characteristic = characteristic;
this.isConnected = true;
this.isConnecting = false;
});
characteristic.value.listen((value) {
print("Received: ${String.fromCharCodes(value)}");
});
break;
}
}
if (characteristic != null) {
break;
}
}
}
void send() {
String data = textEditingController.text;
if (characteristic != null && data.isNotEmpty) {
characteristic!.write(data.codeUnits);
}
}
}
```
上面依赖控件中,`flutter_blue`用于蓝牙的操作,`cupertino_icons`用于展示iOS风格的图标。请在`pubspec.yaml`文件中添加以下依赖:
```yaml
dependencies:
flutter_blue: ^0.8.1
cupertino_icons: ^1.0.2
```
相关推荐
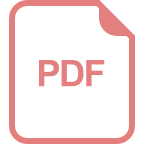
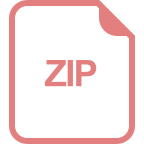
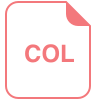
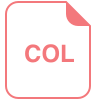
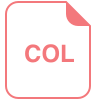
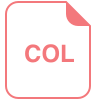
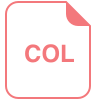









