x = list(range(-T, T+1)) y = [w.count(a) for a in x]
时间: 2024-05-26 10:17:28 浏览: 16
这段代码的作用是统计列表 w 中每个元素在区间 [-T, T] 内出现的次数,并将统计结果存储在列表 y 中。
具体来说,首先创建了一个列表 x,其中包含了从 -T 到 T 的所有整数。然后使用列表推导式计算了列表 y,对于 x 中的每个元素 a,使用 count 方法统计其在列表 w 中出现的次数,并将结果存储在 y 中。最终 y 中的元素顺序与 x 中的元素顺序相同,分别表示 x 中对应元素在 w 中出现的次数。
相关问题
优化这段代码h_now = np.zeros(h) h_list = list(h_now) pix = 0 for y in range(h): for x in range(w): if src[y, x, 1] > 0: pix += 1 h_list[y] = pix
这段代码可以进行一些优化,例如:
1. 使用NumPy的切片操作代替循环遍历像素点,以提高效率。
2. 将列表转换为NumPy数组,并使用NumPy的逻辑运算代替条件判断和累加器,以提高效率。
下面是优化后的代码示例:
```python
import numpy as np
# 假设src是一个三通道的图像数组,其中绿色通道存储了高度信息
h, w, _ = src.shape
# 使用切片操作获取绿色通道的像素值,计算像素总数
pix = np.count_nonzero(src[:, :, 1])
# 将绿色通道的像素值转换为一维数组,统计每行像素数量
h_list = np.sum(src[:, :, 1] > 0, axis=1)
# 将一维数组转换为二维数组,以便后续处理
h_list = h_list.reshape((-1, 1))
# 将二维数组转换为浮点数类型,以便进行除法运算
h_list = h_list.astype(np.float32)
# 将每行像素数量除以像素总数,得到每行的高度比例
h_list /= pix
# 将高度比例数组转换为初始高度数组
h_now = h_list * h
```
这段代码使用了NumPy的切片操作、逻辑运算和统计函数,以及数组广播和类型转换等技巧,以提高代码执行效率。
用python修改以下代码使其能正确运行:# 定义维特比算法 def viterbi(obs, states, start_p, trans_p, emit_p): V = [{}] path = {} for y in states: V[0][y] = start_p[y] * emit_p[y].get(obs[0], 0) path[y] = [y] for t in range(1, len(obs)): V.append({}) newpath = {} for y in states: (prob, state) = max([(V[t-1][y0] * trans_p[y0].get(y, 0) * emit_p[y].get(obs[t], 0), y0) for y0 in states if V[t-1][y0] > 0]) V[t][y] = prob newpath[y] = path[state] + [y] path = newpath (prob, state) = max([(V[len(obs)-1][y], y) for y in states]) return prob, path[state] # 对测试集进行词性标注并计算准确率 total_count = 0 correct_count = 0 for word, pos in test_words: if word in word_pos_prob.get(pos, {}): obs = [word] states = list(pos_count.keys()) start_p = pos_init_prob trans_p = pos_trans_prob emit_p = word_pos_prob[pos] prob, path = viterbi(obs, states, start_p, trans_p, emit_p) if path[pos][0] == pos: correct_count += 1 total_count += 1 accuracy = correct_count / total_count print('Accuracy: {}'.format(accuracy))
# 定义维特比算法
def viterbi(obs, states, start_p, trans_p, emit_p):
V = [{}]
path = {}
for y in states:
V[0][y] = start_p[y] * emit_p[y].get(obs[0], 0)
path[y] = [y]
for t in range(1, len(obs)):
V.append({})
newpath = {}
for y in states:
(prob, state) = max([(V[t-1][y0] * trans_p[y0].get(y, 0) * emit_p[y].get(obs[t], 0), y0) for y0 in states if V[t-1][y0] > 0])
V[t][y] = prob
newpath[y] = path[state] + [y]
path = newpath
(prob, state) = max([(V[len(obs)-1][y], y) for y in states])
return prob, path[state]
# 对测试集进行词性标注并计算准确率
total_count = 0
correct_count = 0
for word, pos in test_words:
if word in word_pos_prob.get(pos, {}):
obs = [word]
states = list(pos_count.keys())
start_p = pos_init_prob
trans_p = pos_trans_prob
emit_p = word_pos_prob[pos]
prob, path = viterbi(obs, states, start_p, trans_p, emit_p)
if path[pos][0] == pos:
correct_count += 1
total_count += 1
accuracy = correct_count / total_count
print('Accuracy: {}'.format(accuracy))
相关推荐
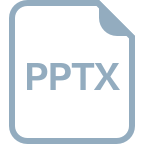
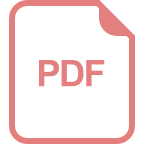
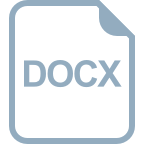













