hc05.c文件的编写过程
时间: 2024-02-16 12:02:48 浏览: 30
编写 HC-05 蓝牙模块的驱动程序 hc05.c 的过程一般如下:
1. 定义头文件包含:
```
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdbool.h>
#include <errno.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/select.h>
#include <sys/time.h>
#include <time.h>
```
2. 定义 HC-05 蓝牙模块的基本参数,如串口号、波特率、数据位、停止位、校验位等。
```
#define DEV_NAME "/dev/ttyS1"
#define BAUD_RATE B9600
#define DATA_BITS CS8
#define STOP_BITS 1
#define PARITY None
```
3. 定义 HC-05 蓝牙模块的初始化函数,包括串口的打开和设置等。
```
int hc05_init(void)
{
int fd;
struct termios opt;
fd = open(DEV_NAME, O_RDWR | O_NOCTTY);
if (fd < 0) {
printf("open device error!\n");
return fd;
}
tcgetattr(fd, &opt);
opt.c_cflag = BAUD_RATE | DATA_BITS | STOP_BITS | PARITY;
opt.c_iflag = IGNPAR;
opt.c_oflag = 0;
opt.c_lflag = 0;
tcflush(fd, TCIFLUSH);
if (tcsetattr(fd, TCSANOW, &opt) != 0) {
printf("set device parameters error!\n");
close(fd);
return -1;
}
return fd;
}
```
4. 定义 HC-05 蓝牙模块的发送函数,将数据通过串口发送出去。
```
int hc05_send(int fd, const char *buf, int len)
{
int ret = 0;
ret = write(fd, buf, len);
if (ret < 0) {
printf("send data error!\n");
return -1;
}
return ret;
}
```
5. 定义 HC-05 蓝牙模块的接收函数,从串口接收数据。
```
int hc05_recv(int fd, char *buf, int len)
{
int ret = 0;
ret = read(fd, buf, len);
if (ret < 0) {
printf("recv data error!\n");
return -1;
}
return ret;
}
```
6. 在主函数中调用 HC-05 蓝牙模块的初始化函数,打开串口,并设置参数。然后调用发送函数和接收函数,进行数据的发送和接收。
```
int main(void)
{
int fd;
char buf[1024];
fd = hc05_init();
if (fd < 0) {
printf("init device error!\n");
return -1;
}
hc05_send(fd, "Hello, HC-05!\n", strlen("Hello, HC-05!\n"));
memset(buf, 0, sizeof(buf));
hc05_recv(fd, buf, sizeof(buf));
printf("recv data: %s\n", buf);
close(fd);
return 0;
}
```
以上就是 HC-05 蓝牙模块的驱动程序 hc05.c 的编写过程。
相关推荐
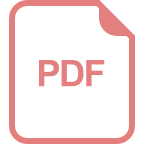
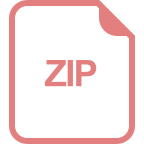
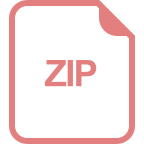















