使用visual studio2019写C语言代码,用中缀法完成四则运算表达式求解(要求手动输入原始表达式后输出计算结果)。
时间: 2024-04-01 07:31:42 浏览: 71
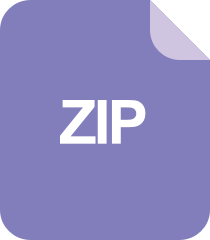
c语言简易计算器实现中缀表达式四则混合运算
好的,以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100 // 定义栈的最大长度
// 定义栈结构体
typedef struct
{
int data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void InitStack(Stack *s)
{
s->top = -1;
}
// 判断栈是否为空
int IsEmpty(Stack *s)
{
return s->top == -1;
}
// 判断栈是否已满
int IsFull(Stack *s)
{
return s->top == MAXSIZE - 1;
}
// 入栈
void Push(Stack *s, int x)
{
if (IsFull(s))
{
printf("Stack is full.\n");
exit(0);
}
s->data[++s->top] = x;
}
// 出栈
int Pop(Stack *s)
{
if (IsEmpty(s))
{
printf("Stack is empty.\n");
exit(0);
}
return s->data[s->top--];
}
// 获取栈顶元素
int GetTop(Stack *s)
{
if (IsEmpty(s))
{
printf("Stack is empty.\n");
exit(0);
}
return s->data[s->top];
}
// 判断是否为运算符
int IsOperator(char ch)
{
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
// 获取运算符的优先级
int GetPriority(char ch)
{
switch (ch)
{
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 中缀表达式转换为后缀表达式
void InfixToPostfix(char *infix, char *postfix)
{
Stack s;
InitStack(&s);
int i = 0;
int j = 0;
while (infix[i] != '\0')
{
if (infix[i] >= '0' && infix[i] <= '9')
{
postfix[j++] = infix[i++];
while (infix[i] >= '0' && infix[i] <= '9')
{
postfix[j++] = infix[i++];
}
postfix[j++] = ' ';
}
else if (IsOperator(infix[i]))
{
while (!IsEmpty(&s) && GetPriority(GetTop(&s)) >= GetPriority(infix[i]))
{
postfix[j++] = Pop(&s);
postfix[j++] = ' ';
}
Push(&s, infix[i++]);
}
else if (infix[i] == '(')
{
Push(&s, infix[i++]);
}
else if (infix[i] == ')')
{
while (GetTop(&s) != '(')
{
postfix[j++] = Pop(&s);
postfix[j++] = ' ';
}
Pop(&s);
i++;
}
else
{
i++;
}
}
while (!IsEmpty(&s))
{
postfix[j++] = Pop(&s);
postfix[j++] = ' ';
}
postfix[j] = '\0';
}
// 计算后缀表达式的值
int EvaluatePostfix(char *postfix)
{
Stack s;
InitStack(&s);
int i = 0;
while (postfix[i] != '\0')
{
if (postfix[i] >= '0' && postfix[i] <= '9')
{
int num = 0;
while (postfix[i] >= '0' && postfix[i] <= '9')
{
num = num * 10 + postfix[i] - '0';
i++;
}
Push(&s, num);
}
else if (postfix[i] == '+')
{
int b = Pop(&s);
int a = Pop(&s);
Push(&s, a + b);
i++;
}
else if (postfix[i] == '-')
{
int b = Pop(&s);
int a = Pop(&s);
Push(&s, a - b);
i++;
}
else if (postfix[i] == '*')
{
int b = Pop(&s);
int a = Pop(&s);
Push(&s, a * b);
i++;
}
else if (postfix[i] == '/')
{
int b = Pop(&s);
int a = Pop(&s);
Push(&s, a / b);
i++;
}
else
{
i++;
}
}
return Pop(&s);
}
int main()
{
char infix[MAXSIZE];
char postfix[MAXSIZE];
printf("请输入中缀表达式:");
gets(infix);
InfixToPostfix(infix, postfix);
printf("后缀表达式为:%s\n", postfix);
int result = EvaluatePostfix(postfix);
printf("计算结果为:%d\n", result);
return 0;
}
```
输入中缀表达式后,程序会自动将其转换为后缀表达式,并输出计算结果。
阅读全文
相关推荐

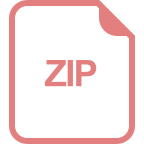













