用C++编程 任务描述 设计两个类:Rectangle(矩形)和Calculator(计算器)。 Rectangle类具有以下成员: 私有数据成员:length(长度),width(宽度) Calculator类具有以下成员: 公有成员函数:calculateArea(),接受一个Rectangle对象作为参数,调用Rectangle对象的私有length和width来计算矩形的面积并显示出来 要求: 将Calculator类声明为Rectangle类的友元类,以便可以访问Rectangle类的私有成员 在主函数中,创建一个Rectangle对象,并使用Calculator类的calculateArea()函数来计算并显示矩形的面积 输入格式为:length width,如:5.2 3.1 输出格式为:The area of the rectangle is 16.12. 提示:使用友元关键字来实现Calculator类对Rectangle类的访问权限。 样例输入:5.2 3.1 样例输出:The area of the rectangle is 16.12. 样例输入:15.2 13.1 样例输出:The area of the rectangle is 199.12. 样例输入:25.2 23.1 样例输出:The area of the rectangle is 582.12.
时间: 2024-02-01 19:16:59 浏览: 24
以下是代码实现:
```cpp
#include <iostream>
using namespace std;
class Rectangle; // 先声明 Rectangle 类
class Calculator {
public:
void calculateArea(Rectangle r) { // 计算矩形面积
double area = r.length * r.width;
cout << "The area of the rectangle is " << area << "." << endl;
}
};
class Rectangle {
private:
double length;
double width;
friend class Calculator; // 声明 Calculator 类为友元类
public:
Rectangle(double l, double w) : length(l), width(w) {}
};
int main() {
double l, w;
cin >> l >> w;
Rectangle r(l, w);
Calculator c;
c.calculateArea(r);
return 0;
}
```
代码思路:
1. 定义 Rectangle 类,其中包含私有数据成员 length 和 width。
2. 定义 Calculator 类,其中包含公有成员函数 calculateArea(),接受一个 Rectangle 对象作为参数,计算矩形的面积并显示出来。
3. 在 Rectangle 类的定义中,声明 Calculator 类为友元类,以便可以访问 Rectangle 类的私有成员。
4. 在主函数中,创建一个 Rectangle 对象,并使用 Calculator 类的 calculateArea() 函数来计算并显示矩形的面积。
需要注意的是,我们在上面的代码中使用了类的友元关键字 friend,来实现对 Rectangle 类的私有成员的访问权限。
相关推荐
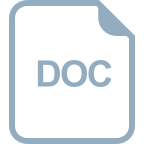
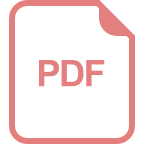
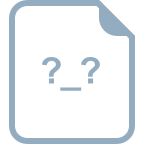














